Table of Contents
Java provides several ways to initialize a HashMap directly, making your code more concise and readable. In this blog post, we’ll explore different methods to create and populate a HashMap in one go using Java.
1. Using Map.of() (Java 9+)
For small, immutable maps, you can use the Map.of()
method:
Map<String, Integer> map = Map.of( "Apple", 1, "Banana", 2, "Cherry", 3 );
This method is convenient but limited to a maximum of 10 key-value pairs.
2. Using Map.ofEntries() (Java 9+)
For larger immutable maps, use Map.ofEntries()
:
Map<String, Integer> map = Map.ofEntries( Map.entry("Apple", 1), Map.entry("Banana", 2), Map.entry("Cherry", 3), Map.entry("Date", 4) );
This method can handle any number of entries.
3. Using the Double Brace Initialization (Not Recommended)
While it works, this method is generally discouraged due to potential memory leaks:
Map<String, Integer> map = new HashMap<>() {{ put("Apple", 1); put("Banana", 2); put("Cherry", 3); }};
4. Using Stream API (Java 8+)
For more complex initialization scenarios, you can use the Stream API:
Map<String, Integer> map = Stream.of( new AbstractMap.SimpleEntry<>("Apple", 1), new AbstractMap.SimpleEntry<>("Banana", 2), new AbstractMap.SimpleEntry<>("Cherry", 3) ).collect(Collectors.toMap( Map.Entry::getKey, Map.Entry::getValue ));
5. Using Map.copyOf() (Java 10+)
To create an immutable copy of an existing map:
Map<String, Integer> originalMap = new HashMap<>(); originalMap.put("Apple", 1); originalMap.put("Banana", 2); Map<String, Integer> immutableMap = Map.copyOf(originalMap);
6. Mutable Initialization with Java 17’s Text Blocks
Java 17 introduced text blocks, which can be parsed to initialize a HashMap:
String jsonMap = """ { "Apple": 1, "Banana": 2, "Cherry": 3 } """; ObjectMapper mapper = new ObjectMapper(); Map<String, Integer> map = mapper.readValue(jsonMap, new TypeReference<HashMap<String, Integer>>() {});
Note: This method requires the Jackson library.
Conclusion
Java 17 offers multiple ways to initialize a HashMap directly. Choose the method that best fits your use case, considering factors like mutability, number of entries, and readability. Remember that methods like Map.of()
and Map.ofEntries()
create immutable maps, while others allow for mutable maps.
Happy coding!
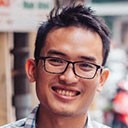
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.