Table of Contents
- 1 1. Understand System Architecture
- 2 2. Learn Communication Patterns
- 3 3. Data Consistency Awareness
- 4 4. Error Handling and Fault Tolerance
- 5 5. Service Discovery
- 6 6. Efficient Data Handling
- 7 7. Understand CAP Theorem Trade-offs
- 8 8. Concurrency Management
- 9 9. Message Passing and Asynchronous Processing
- 10 10. Security Practices
- 11 11. Understand Data Serialization Formats
- 12 12. Testing Distributed Systems
- 13 13. Logging and Observability
- 14 14. Performance Optimization
- 15 15. Resource and Rate Management
- 16 16. Learn Deployment and CI/CD Practices
- 17 17. Handle Service Versioning
- 18 18. Understand Time and Clock Synchronization Issues
- 19 19. Collaborate with Architects and Other Developers
- 20 20. Documentation and Knowledge Sharing
1. Understand System Architecture
- Definition: Get familiar with the system’s architecture and how components interact.
- Key Concepts: Microservices, service boundaries, APIs, and system dependencies.
2. Learn Communication Patterns
- Definition: Understand how services communicate in a distributed system.
- Techniques:
- Synchronous (HTTP, gRPC) for immediate responses.
- Asynchronous (message queues, event streams) for decoupling and resilience.
3. Data Consistency Awareness
- Definition: Be aware of how data consistency is handled.
- Key Concepts:
- Strong consistency: Immediate synchronization.
- Eventual consistency: Updates propagate over time.
- Implications: Design code to handle stale or out-of-sync data.
4. Error Handling and Fault Tolerance
- Definition: Expect and handle failures in network, services, and data.
- Strategies:
- Implement retries with exponential backoff.
- Use circuit breakers to prevent cascading failures.
- Handle partial failures gracefully.
5. Service Discovery
- Definition: Dynamically find services within the distributed system.
- Tools: Service registries like Consul, Zookeeper, or built-in Kubernetes discovery.
6. Efficient Data Handling
- Definition: Optimize data processing and storage for distributed environments.
- Techniques:
- Use distributed caches (e.g., Redis) to reduce latency.
- Partition (shard) data to distribute load across nodes.
7. Understand CAP Theorem Trade-offs
- Definition: Distributed systems can prioritize only two of Consistency, Availability, or Partition Tolerance.
- Developer Focus: Recognize which trade-offs your system has made and design accordingly.
8. Concurrency Management
- Definition: Handle concurrent operations safely and efficiently.
- Strategies:
- Avoid race conditions by using optimistic or pessimistic locks.
- Implement idempotent operations to prevent issues from duplicate requests.
9. Message Passing and Asynchronous Processing
- Definition: Use messaging for communication between decoupled services.
- Examples:
- RabbitMQ, Kafka, or AWS SNS/SQS for message queues.
- Event-driven programming for scalability and fault isolation.
10. Security Practices
- Definition: Protect data and communication across distributed components.
- Best Practices:
- Secure APIs using OAuth, JWT, or API keys.
- Encrypt data in transit and at rest (e.g., TLS, encryption libraries).
- Validate input to prevent injection attacks.
11. Understand Data Serialization Formats
- Definition: Ensure services can efficiently exchange data.
- Common Formats: JSON, Protocol Buffers (Protobuf), Avro.
- Developer Focus: Use compact, schema-defined formats (e.g., Protobuf) for high performance.
12. Testing Distributed Systems
- Definition: Ensure that your system behaves correctly under distributed conditions.
- Types of Tests:
- Unit tests for individual components.
- Integration tests for inter-service communication.
- Chaos testing (e.g., with Chaos Monkey) to simulate failures.
13. Logging and Observability
- Definition: Provide visibility into the system’s behavior for troubleshooting.
- Developer Tasks:
- Implement structured logging.
- Use distributed tracing (e.g., Jaeger, OpenTelemetry) to follow requests across services.
- Collect metrics for performance monitoring.
14. Performance Optimization
- Definition: Improve system performance by reducing bottlenecks.
- Developer Tips:
- Use caching to reduce database queries.
- Minimize network overhead by batching or compressing requests.
- Profile services to identify slow operations.
15. Resource and Rate Management
- Definition: Prevent resource exhaustion and maintain service stability.
- Techniques:
- Implement rate limiting and backpressure.
- Use resource quotas to prevent overload.
16. Learn Deployment and CI/CD Practices
- Definition: Understand how distributed systems are deployed and updated.
- Key Concepts:
- Blue-green deployments to minimize downtime.
- Canary releases to gradually test changes in production.
- Automated CI/CD pipelines to ensure consistency.
17. Handle Service Versioning
- Definition: Manage changes in services and APIs without breaking compatibility.
- Developer Strategies:
- Use versioned APIs (e.g., /v1/service).
- Implement backward-compatible changes.
- Deprecate old versions in a planned manner.
18. Understand Time and Clock Synchronization Issues
- Definition: Be aware of how time discrepancies can affect distributed systems.
- Challenges:
- Use NTP for clock synchronization.
- Design systems to tolerate clock skew (e.g., use timestamps cautiously).
19. Collaborate with Architects and Other Developers
- Definition: Work closely with stakeholders to align on system design and functionality.
- Focus Areas:
- Clarify service contracts and data formats.
- Participate in design reviews and architectural discussions.
20. Documentation and Knowledge Sharing
- Definition: Maintain documentation to help others understand and maintain the system.
- Developer Focus:
- Document APIs, endpoints, and service dependencies.
- Write clear comments and README files.
- Share lessons learned from debugging and production incidents.
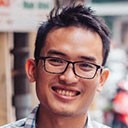
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.