Table of Contents
Overview
Recently I struggled with building my new app with Vite+React for production. The issue was something to do with _global in the recent axios versions.
When running npm run build
file: /Users/mac/Documents/projects/video-pipeline-ui/node_modules/axios/lib/utils.js:681:6 679: 680: return postMessageSupported ? ((token, callbacks) => { 681: _global.addEventListener("message", ({source, data}) => { ^ 682: if (source === _global && data === token) { 683: callbacks.length && callbacks.shift()(); error during build: SyntaxError: Unexpected token (681:6) in /Users/mac/Documents/projects/video-pipeline-ui/node_modules/axios/lib/utils.js at pp$4.raise (file:///Users/mac/Documents/projects/video-pipeline-ui/node_modules/rollup/dist/es/shared/rollup.js:19420:13) at pp$9.unexpected (file:///Users/mac/Documents/projects/video-pipeline-ui/node_modules/rollup/dist/es/shared/rollup.js:16714:8) at pp$5.parseExprAtom (file:///Users/mac/Documents/projects/video-pipeline-ui/node_modules/rollup/dist/es/shared/rollup.js:18795:10) at pp$5.parseExprSubscripts (file:///Users/mac/Documents/projects/video-pipeline-ui/node_modules/rollup/dist/es/shared/rollup.js:18587:19) at pp$5.parseMaybeUnary (file:///Users/mac/Documents/projects/video-pipeline-ui/node_modules/rollup/dist/es/shared/rollup.js:18553:17) at pp$5.parseExprOps (file:///Users/mac/Documents/projects/video-pipeline-ui/node_modules/rollup/dist/es/shared/rollup.js:18480:19)
So here is how I fixed that (after searching for solutions on Google)
How to fix axios error when running vite build
The fix is quite simple, I added this
define: { global: 'globalThis'}, optimizeDeps: { esbuildOptions: { // Node.js global to browser globalThis define: { global: 'globalThis', }, }, },
To vite.config.ts
And I was able to build.
The whole file looks like this:
import path from "path" import react from "@vitejs/plugin-react" import {defineConfig} from "vite" export default defineConfig(() => { return { base: "/", plugins: [react()], resolve: { alias: { "@": path.resolve(__dirname, "./src"), }, }, define: { global: 'globalThis'}, optimizeDeps: { esbuildOptions: { // Node.js global to browser globalThis define: { global: 'globalThis', }, }, }, } })
Now it’s time for dockerfile and nginx
Adding Dockerfile and nginx.conf
Here are my Dockerfile and nginx.conf, the process is quite straightforward
server { listen 80; server_name localhost; gzip on; gzip_types text/plain application/xml; gzip_proxied no-cache no-store private expired auth; gzip_min_length 1000; root /usr/share/nginx/html; index index.html; location / { try_files $uri $uri/ /index.html; } error_page 500 502 503 504 /50x.html; location = /50x.html { root /usr/share/nginx/html; } }
FROM nginx:1.23.1-alpine COPY nginx.conf /etc/nginx/conf.d/default.conf COPY /dist /usr/share/nginx/html
Now, you can run npm run build
and then docker build -t your_image_nage .
To build the image.
Conclusion
That’s how I fixed the issue with axios when built with vite and the nginx.conf & dockerfile. Hope that helps.
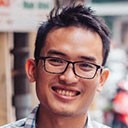
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.