Table of Contents
Mastering system design is about learning to balance complex trade-offs and make reasoned architectural decisions. As software architect Mark Richards famously said, “Everything in software architecture is a trade-off.” (The First Law of Software Architecture: Understanding Trade-offs – DEV Community) This multi-phase roadmap will guide a web developer (with mid-scale SaaS experience) through a deep dive into system design, with a focus on real-time systems, high-throughput APIs, and event-driven architectures. Each phase includes clear goals, hands-on projects, core topics, and high-quality resources (books, case studies, open-source projects, videos, papers). We emphasize project-based learning, exposure to real-world engineering practices, and constant reflection on the reasoning and trade-offs behind design decisions.
Note: Allocate ~8 hours per week for these activities. Keep a learning journal to record insights and trade-offs encountered. Regularly discuss what you build/learn with peers or mentors for feedback.
Phase 1: Foundation – Solidifying Core Concepts
In the Foundation phase, you’ll build a strong base in fundamental concepts of scalable system design. The goal is to ensure you understand the building blocks and can reason about basic trade-offs (latency vs throughput, consistency vs availability, etc.) (System Design Roadmap: A Step-by-Step Guide to Mastering System Design – DEV Community) (15 System design tradeoffs for Software Developer Interviews – DEV Community). You will also get introduced to real-time and event-driven basics in preparation for deeper dives later.
Goals
- Grasp fundamental concepts – e.g. how web systems work (client-server model, HTTP), how data is stored and retrieved (SQL vs NoSQL) (15 System design tradeoffs for Software Developer Interviews – DEV Community), and how networks and caches improve performance.
- Understand key trade-offs – latency vs throughput (15 System design tradeoffs for Software Developer Interviews – DEV Community), vertical vs horizontal scaling (15 System design tradeoffs for Software Developer Interviews – DEV Community), consistency vs availability (CAP theorem) (System Design Roadmap: A Step-by-Step Guide to Mastering System Design – DEV Community), stateless vs stateful services (15 System design tradeoffs for Software Developer Interviews – DEV Community), etc., and when to favor one side or the other.
- Learn basic architecture patterns – layered architecture, load balancing, caching, database sharding/replication basics, and message queues.
- Familiarize with real-time & EDA basics – what constitutes a real-time system (e.g. WebSockets vs polling) and what is an event-driven architecture (producers, consumers, message brokers) at a high level.
- Establish learning habits – set up a system design journal, and a routine for reading and hands-on practice 8 hours weekly.
Core Topics to Cover
- Scalability 101: Client-server model; the difference between scaling up vs scaling out (15 System design tradeoffs for Software Developer Interviews – DEV Community); introduction to cloud infrastructure (servers, load balancers, CDNs).
- Performance Basics: Latency vs throughput trade-offs (15 System design tradeoffs for Software Developer Interviews – DEV Community); how to measure response time; the impact of network latency and data size on performance.
- Reliability Basics: Redundancy and failover concepts; CAP theorem (Consistency, Availability, Partition Tolerance) and understanding strong vs eventual consistency (15 System design tradeoffs for Software Developer Interviews – DEV Community).
- Data Management Fundamentals: SQL vs NoSQL databases (when to use each) (15 System design tradeoffs for Software Developer Interviews – DEV Community); indexing and query optimization; basics of data replication and sharding.
- Caching & CDN: How caching works (memory vs disk cache, TTL); cache aside vs write-through strategies (15 System design tradeoffs for Software Developer Interviews – DEV Community); using CDNs for static content (System Design Roadmap: A Step-by-Step Guide to Mastering System Design – DEV Community).
- Stateful vs Stateless Services: Benefits of statelessness for scaling vs stateful design for performance (e.g. sticky sessions, in-memory state) (15 System design tradeoffs for Software Developer Interviews – DEV Community).
- Communication Patterns: Synchronous (request/response HTTP) vs asynchronous messaging; introduction to messaging systems (e.g. simple queue, pub/sub) and when async processing is beneficial.
- Real-Time Intro: Long polling vs WebSockets (trade-offs in complexity and performance) (15 System design tradeoffs for Software Developer Interviews – DEV Community); basics of pushing updates to clients in real-time applications.
- Event-Driven Intro: Definition of events, producers & consumers; simple event-driven use-case (e.g. user registration event triggers a welcome email). Focus on the conceptual benefit of loose coupling and eventual consistency in exchange for complexity.
Projects to Build (Hands-on Practice)
Put theory into practice with small projects. The aim is to expose yourself to real systems behavior and force yourself to consider design choices:
- 1. Simple Real-Time Notification Service: Build a tiny web app that pushes updates to connected clients in real time. For example, create a server that sends a “tick” message to all clients every second or broadcasts chat messages. Use WebSockets (or an abstraction like Socket.IO) to maintain a persistent connection. This project will teach you how a basic real-time channel works (handshake, messaging, broadcast). Observe the latency – messages should appear near-instantly. Trade-offs to consider: long polling vs WebSocket (implement a basic long-poll endpoint to compare and note the higher latency/overhead of HTTP long polling) (15 System design tradeoffs for Software Developer Interviews – DEV Community). Document why persistent connections are beneficial for low-latency updates.
- 2. Load-Balanced App with Caching: Take a simple API (e.g., a read-heavy endpoint like “get article by ID”) and improve its throughput. Set up two instances of the API server and put Nginx in front to round-robin between them (simulate a load balancer). Introduce a cache (e.g., Redis or an in-memory cache) for the API responses. Use a tool (like
ab
, JMeter, or k6) to send concurrent requests and measure: throughput (requests/sec) and latency (response time) with and without caching and load balancing. This will give you practical experience with horizontal scaling and caching benefits (System Design Roadmap: A Step-by-Step Guide to Mastering System Design – DEV Community) (System Design Roadmap: A Step-by-Step Guide to Mastering System Design – DEV Community). Trade-offs to consider: consistency (serving stale data from cache vs always hitting DB), complexity (added Nginx and Redis) vs performance gain. Ensure you can explain how the load balancer improves capacity and how caching reduces database load. - 3. Asynchronous Processing Demo: Implement a basic event-driven workflow in your app. For example, when a user uploads a file, instead of processing it synchronously, place a message on a queue (use a simple message broker like RabbitMQ, Redis Pub/Sub, or even an in-memory queue for demo). Have a separate worker process subscribe to the queue and perform the processing (e.g., image resizing or sending a confirmation email). The web request immediately returns a success response, offloading work to the background. This project introduces you to event-driven design and message queues. Trade-offs to consider: throughput vs simplicity – adding a queue increases system complexity but allows handling higher load by smoothing spikes and freeing up web threads quickly. Note how this introduces eventual consistency (the result of processing isn’t immediate). Consider what happens if the worker fails (need for retry or DLQ concept, which you’ll explore later).
Each mini-project above is manageable in a few hours. Focus on reasoning: after each project, write a short reflection on what trade-offs you encountered (e.g., “WebSockets vs polling for real-time updates – polling was easier but had higher latency and wasted resources, whereas WebSockets were more efficient but require stateful connections (15 System design tradeoffs for Software Developer Interviews – DEV Community).”). This reflection habit will reinforce your learning.
High-Quality Resources (Foundation)
Leverage these resources to solidify concepts and learn from experts:
- Book – Designing Data-Intensive Applications by Martin Kleppmann: A comprehensive guide to building scalable, reliable data systems, covering storage engines, distributed systems, and trade-offs in detail (System Design Roadmap: A Step-by-Step Guide to Mastering System Design – DEV Community). Approach: Start reading in this phase (the early chapters on data models, storage, and replication align with foundation topics). Take notes on trade-off discussions (e.g., relational vs NoSQL, consistency models). This book will be a reference throughout your journey.
- The System Design Primer (GitHub) – An open-source repository of system design basics and interview questions. Review the sections on scalability, performance, database, and caching to reinforce fundamentals (e.g., the primer’s explanation of performance vs scalability trade-off in architecture) (README.md – donnemartin/system-design-primer – GitHub) (Seeking advice on DE System Design : r/dataengineering – Reddit). It’s a great checklist of topics to ensure you haven’t missed anything.
- Educative Grokking System Design (or similar course) – If you prefer a structured interactive course, Grokking has scenarios that walk through fundamental design problems. Focus on the early modules (load balancers, caches, key-value store design) to see practical applications of foundational concepts.
- Trade-Off Articles: “15 System Design Tradeoffs” (15 System design tradeoffs for Software Developer Interviews – DEV Community) – read this dev.to article (or ByteByteGo newsletter) that enumerates common trade-off pairs (e.g., Stateful vs Stateless, SQL vs NoSQL, Strong vs Eventual consistency). This will reinforce your ability to articulate pros/cons. Similarly, “The First Law of Software Architecture” by DevCorner is a short read emphasizing why understanding trade-offs is the essence of architecture (The First Law of Software Architecture: Understanding Trade-offs – DEV Community) (The First Law of Software Architecture: Understanding Trade-offs – DEV Community).
- Video – System Design Basics (TechTalk or university lectures): Find a high-level talk or lecture series on system design fundamentals (for example, Stanford’s CS244b or MIT’s 6.824 have introductory lectures on distributed systems theory – if available online). Watching an expert explain concepts like CAP theorem, replication, or caching will deepen your conceptual understanding.
- Real-World Case Study (Light): Read “Scalable Web Architecture and Distributed Systems” (an older but classic blog post by Kate Matsudaira) or the HighScalability summary of it. It walks through how a hypothetical website scales from one server to millions of users, touching on load balancing, caching, database scaling, etc. This narrative solidifies how foundation concepts come into play as load grows.
Optional Deep Dives (Foundation): If you have extra time or curiosity in this phase, consider exploring:
- Distributed Systems Theory: The free e-book “Distributed Systems for Fun and Profit” (M. K. Tacke) for a gentle theoretical background on consensus, time in distributed systems, etc.
- Networking Under the Hood: Understand what happens at the TCP/UDP level (e.g., read about the TCP handshake, how WebSockets keep a TCP connection) – this can help when tuning real-time systems later. The book “High Performance Browser Networking” (Ilya Grigorik) has accessible chapters on WebSockets and network fundamentals.
- Language-specific Concurrency: If you primarily use one programming language, take time to learn its concurrency model and frameworks (e.g., Node.js event loop, Python asyncio, Java threads & executors). Knowing how to write efficient concurrent code will help in implementing high-throughput services.
Validate Your Understanding
At the end of Phase 1, test your foundation knowledge:
- Concept Explanations: Try to explain key concepts (CAP theorem, load balancing, etc.) in simple terms to a colleague or in a short memo. If you can clearly explain why, say, a cache improves throughput or what trade-off CAP imposes, you’re on the right track.
- Mock Interview (Fundamentals): Design a simple system with someone (or alone on paper) – e.g., “Design a URL Shortener” or “Design a simple chat server for 100k users.” Focus on using foundation concepts: talk about using a cache, how to scale reads, etc. Pay attention to justifying each decision with trade-offs (for instance, “I’ll use a NoSQL store for URLs for scalability and simplicity of key-value access, trading off some relational capabilities”). This helps transition from theory to design rationale.
- Peer Review: If possible, have an experienced friend or mentor review your project code/architecture. For example, walk them through your load-balanced app setup and see if they spot any improvements or ask “Why did you choose this approach?” Their questions will highlight if you understand the reasoning.
- Flashcards/Anki: Create flashcards for core concepts (e.g., definitions of throughput, eventually consistent, etc.) and ensure you can recall and apply them quickly.
By the end of the Foundation phase, you should feel comfortable with the vocabulary of system design and have a mental toolbox of basic techniques. You’ll also appreciate that every choice (database, cache, protocol) comes with trade-offs – an essential mindset for the next phases (15 System design tradeoffs for Software Developer Interviews – DEV Community) (The First Law of Software Architecture: Understanding Trade-offs – DEV Community).
Phase 2: Intermediate – Building Real-Time & Event-Driven Systems
The Intermediate phase moves from fundamentals to application. Here you will design and build systems with real-time and event-driven features, and learn to handle moderate scale (think millions of users or requests). The emphasis is on practical projects that mimic real-world scenarios and on learning from industry case studies. By the end of this phase, you should be adept at designing a system with multiple interacting components (e.g., client, API, database, message queue) and understand how to make it scalable and reliable for mid-scale use.
Goals
- Apply fundamentals to real systems: Build and integrate components like WebSocket gateways, message brokers, caching layers, etc., to create real-time and asynchronous functionality in your projects. (https://pages.ably.com/hubfs/the-websocket-handbook.pdf)
- Deepen event-driven architecture knowledge: Understand patterns and components of event-driven systems (producers, consumers, brokers) and their benefits/trade-offs (loose coupling, but added complexity and eventual consistency) (Event-Driven Architecture Roadmap | Deepak Bhardwaj | 13 comments).
- Design high-throughput APIs: Learn techniques to handle high request volumes – such as stateless scaling, connection pooling, efficient data serialization, and rate limiting. Aim to comfortably design an API that could serve ~10k+ requests/sec with low latency.
- Explore microservices and integration: If you’re used to monoliths, get hands-on with splitting service and using events or a pub/sub mechanism for communication. Learn how real-world microservices use queues, topics, and orchestration to work together.
- Trade-off reasoning in practice: When adding each new component or feature, explicitly consider alternatives and justify choices. E.g., “Should I use polling or WebSockets for this feature? Why choose one over the other given my requirements?” This will become natural with practice.
- Exposure to real-world designs: Study how companies build real-time, high-scale systems. Learn from case studies – both their successes and the trade-offs they made (e.g., Slack’s decision to keep state in memory for speed (Real-Time Messaging Architecture at Slack – InfoQ), or WhatsApp’s choice of Erlang for massive concurrency with a small team (14 Case Studies: Master System Design in a Month – DEV Community)). These will inspire and inform your own designs.
Core Topics to Cover
- Message Brokers & Pub/Sub: Learn how messaging systems like Apache Kafka, RabbitMQ, or cloud equivalents (AWS SNS/SQS, Google Pub/Sub) work. Key concepts: topics, partitions, message offset, at-least-once vs at-most-once delivery, consumer groups. Understand how brokers enable decoupling and horizontal scaling in event-driven systems (Event-Driven Architecture Roadmap | Deepak Bhardwaj | 13 comments).
- Event-Driven Patterns: Study basic patterns like event notification (trigger an email or cache update on an event) vs event-carried state transfer (event contains the data needed) vs event sourcing (log every state change) – know the differences. Introduce the concept of CQRS (Command Query Responsibility Segregation), where writes and reads are separated (often with events updating read models). At this stage, just grasp conceptually how event sourcing and CQRS ensure scalability and eventual consistency (Roadmap to Backend Programming Master: Real-Time Data | by Lagu | Medium) (Roadmap to Backend Programming Master: Real-Time Data | by Lagu | Medium), you will implement them in the next phase.
- Real-Time Communication: Dive deeper into WebSockets, Server-Sent Events (SSE), and HTTP/2 or gRPC streaming. Know the pros/cons: e.g., WebSockets offer true bidirectional low-latency comm, SSE are unidirectional but simpler, gRPC streams are efficient but require protobuf (binary protocol) and HTTP/2. Consider use cases for each (chat app vs live sports scores vs IoT updates). Also learn about backpressure in real-time streams (what if client can’t keep up with messages) – e.g., techniques like dropping messages or buffering (ReactiveX concepts) (Roadmap to Backend Programming Master: Real-Time Data | by Lagu | Medium) (Roadmap to Backend Programming Master: Real-Time Data | by Lagu | Medium).
- Microservices & Integration: Understand how to design a system as a set of services. Topics: service boundaries (e.g., user service, order service), synchronous REST calls vs async events between services, API gateways. Learn about API Gateway vs message broker usage (gateway for synchronous APIs, broker for async events) – in practice, many architectures use both (gateway for user requests, events for internal communication). Also cover idempotency and deduplication – crucial for reliability in microservices (e.g., how Stripe’s idempotent APIs ensure no double charges (14 Case Studies: Master System Design in a Month – DEV Community)).
- High-Throughput API Techniques: Explore how to make an API handle heavy load. Topics: connection pooling, thread vs event-loop model (e.g., using Node.js or Golang for handling many concurrent connections efficiently), using binary protocols (gRPC/protobuf) instead of JSON to reduce payload size (trade-off: human-readability vs performance) – for example, Discord found gRPC beneficial for high throughput calls (Key concept of System design going backward – Substack). Study rate limiting algorithms (token bucket, leaky bucket) and implement a simple rate limiter to understand protecting APIs from overload.
- Data Scaling: In the intermediate, implement practical sharding or replication on a small scale. For instance, use a primary-replica database setup and direct reads to replicas to increase throughput. Learn about read consistency issues that arise (stale reads) and how systems like Reddit or Instagram handle read-after-write consistency with slight delays – reinforcing the concept of trade-offs between throughput and consistency.
- Monitoring & Observability Basics: As you build more complex systems, start using simple monitoring: e.g., add logging of request latency, use an open-source tool like Prometheus + Grafana or even simple statsd to collect metrics from your project. Understand what metrics are important (requests/sec, 99th percentile latency, error rate). This will prepare you for observing and tuning systems in the Advanced phase.
Projects to Build (Hands-on Practice)
The projects in this phase are more involved. Aim to produce one or two portfolio-worthy systems that incorporate real-time features, event-driven processing, and can handle higher loads. Here are recommended project ideas:
- 4. Real-Time Chat Service (Case Study: Slack-like Chat): Design and build a simplified chat application with a focus on real-time messaging and scale. Components might include: a WebSocket Gateway server that clients connect to for receiving messages, a backend service for message persistence (e.g., saving to a database), and a publish/subscribe mechanism to distribute messages to all clients in a chat room. For instance, when a user sends a message, the message service writes it to DB (if persistence is needed) and publishes an event (
NewMessage
with content and room ID) to a message broker. The WebSocket Gateway subscribes to relevant channels (topics per chat room) and pushes the message to all connected clients. Technologies: Use Redis Pub/Sub or RabbitMQ (easier setup) as the broker for events, or even serverless WebSocket offerings if curious (like AWS API Gateway WebSocket). Focus on achieving low latency delivery. Simulate scale: Launch multiple gateway instances (to simulate horizontal scaling) and ensure a message from any user still reaches all users (you’ll realize the need for a central pub/sub or coordination – which is why Slack uses a fan-out architecture with channel servers). This project will teach you about stateful vs stateless services: your WebSocket server is likely stateful (holds connections), whereas your message service could be stateless. Discuss the trade-off Slack made by using stateful Channel Servers that keep message history in memory for speed (Real-Time Messaging Architecture at Slack – InfoQ). Validation: Conduct a simple load test – e.g., have 100 clients connect (can script this) and broadcast messages, see how your system holds up. Measure message delivery time. If needed, implement basic backpressure (maybe limit messages per second per client). - 5. Event-Driven Microservice & Analytics Pipeline: Create a mini event-driven architecture with multiple services to see EDA in action. For example, design a user activity tracking system: When a user acts as your app (e.g., makes a purchase or “likes” a post), one service emits an event (e.g., UserLikedPost or PurchaseMade event) to an event stream. Downstream, two different services consume this event: one could update a real-time analytics dashboard (e.g., increment a “likes” count visible on an admin panel via WebSocket), another could perform a secondary action (e.g., send a recommendation update or trigger an email). Use Kafka or RabbitMQ to broker the events (Kafka is more involved but great to learn for streaming at scale; RabbitMQ is simpler for smaller-scale events). What to implement: A producer in your web app that publishes events, a Kafka/Rabbit consumer service that processes events (perhaps aggregates them or writes to a summary table), and optionally a second consumer for another purpose. Also build a simple front-end (or log output) to reflect the processed data (e.g., number of likes today). This pipeline will solidify concepts of event queues, consumer groups, offset (Kafka), or acknowledgments (RabbitMQ). Trade-offs: Note the eventual consistency – the dashboard update is not in real-time with the original action but may be a few seconds delayed. Discuss how this is acceptable for throughput and decoupling reasons. Think about ordering (Kafka maintains order per partition, RabbitMQ doesn’t guarantee global order by default) – if your use-case needed order, how would that influence design? This project also forces you to handle failures: try stopping your consumer and generating events, then restart – with Kafka, the events are retained and will be processed (if committed offsets properly), with RabbitMQ, depending on config, it might have queued them. Understanding this behavior is gold.
- 6. High-Throughput REST API with Caching and DB Sharding: Design an API service for a scenario that expects heavy read traffic – for example, an API to fetch popular content or a product catalog frequently accessed. Implement it with careful optimizations: a read-through cache (Redis) in front of a database, and maybe partition the database or use a read-replica to split the load. Project steps: Populate a database with dummy data (say 1 million product records). Build an API endpoint
/products/top10
that returns the top 10 products. Apply performance tricks: use Redis to cache the result of this query, and expire it every few seconds. If feeling adventurous, set up two database instances each with half the data (shard by some key) or one primary-one secondary (writes to primary, reads from secondary). Perform a load test: ramp up the number of requests and measure when the latency starts rising or errors appear. Tune parameters like thread pool size or use an async framework to see differences in throughput. Goal: Achieve a high number of requests per second with acceptable latency. Document the before/after of each optimization (e.g., “Without cache, DB CPU went high and we hit 200ms latency at 100 req/s; with caching, stayed <50ms even at 500 req/s”). This will teach you practical capacity planning and the effect of each layer on scalability. It also underlines trade-offs: e.g., serving from cache means some requests might get slightly stale data – a conscious decision many real systems make for speed.
By executing these projects, you’ll encounter many real-world challenges: broadcasting to many users (scaling fan-out), handling event ordering and duplication, hot keys in cache, etc. Embrace these as learning opportunities. Where you struggle, try to find how big companies solved similar problems.
Real-World Case Studies & Reading (Intermediate)
To ground your learning in reality, study the architecture of well-known systems, focusing on their real-time and high-throughput aspects and the trade-offs behind them:
- Slack’s Real-Time Messaging Architecture: Read Slack’s engineering blog post “Real-time Messaging at Slack” or the InfoQ summary of it. Key insights: Slack maintains tens of millions of concurrent WebSocket connections and delivers messages worldwide with ~500ms latency by using a custom pub/sub architecture (Real-Time Messaging Architecture at Slack – InfoQ) (Real-Time Messaging Architecture at Slack – InfoQ). They employ stateful Channel Servers that hold chat history in memory, partitioned by consistent hashing (trade-off: stateful for speed vs stateless simplicity) (Real-Time Messaging Architecture at Slack – InfoQ). Note how Envoy is used for load balancing WebSocket connections (Real-Time Messaging Architecture at Slack – InfoQ). This case study shows how real-time collaboration tools achieve scale – by carefully balancing memory usage, data locality, and partitioning to keep latency low.
- WhatsApp’s Scale with Simplicity: WhatsApp supported ~50 billion messages per day for 450M users with only 32 engineers (14 Case Studies: Master System Design in a Month – DEV Community). Research how (several articles enumerate their principles). They chose Erlang for its lightweight concurrency and fault tolerance out of the box, which let a small team manage a huge scale. They kept the system design simple – one kind of server process handling messaging, sharded by phone number. The takeaway: using the right tool (Erlang) and focusing on a narrow problem (messaging) let them avoid complexity and scale vertically to some extent. The trade-off was using a niche technology but it paid off in reliability. Understanding WhatsApp’s approach will broaden your perspective (sometimes simplicity and focus are the ultimate design hacks).
- Uber’s Event-Driven Uber (Real-Time) Platform: Uber’s ride-matching system is a great example of a high-throughput, real-time event-driven system. Read the HighScalability summary of Uber’s dispatch architecture. Uber’s dispatch (matching riders with drivers) is essentially a real-time marketplace processing millions of geolocation events. They set a goal of handling 1 million writes/sec to their geospatial index (driver locations) with many million reads (How Uber Scales Their Real-time Market Platform – High Scalability –). They achieved this with a partitioned in-memory index (sharded by region/city) and a gossip-based consistent hashing system (the Ringpop library) to distribute load and handle node failures (How Uber Scales Their Real-time Market Platform – High Scalability –) (How Uber Scales Their Real-time Market Platform – High Scalability –). Key trade-offs: they chose eventual consistency (using gossip to propagate state) over a strongly consistent centralized system, to gain availability and partition tolerance (an application of CAP theorem under extreme load). Studying Uber will reinforce concepts like sharding, eventual consistency in practice, and using redundant data on the edge (driver phones) for resilience (Uber can fall back to data cached on drivers’ phones if needed (How Uber Scales Their Real-time Market Platform – High Scalability –)!). This is an advanced case, but even at intermediate stage you can appreciate their design choices.
- Additional Cases (optional): Netflix (real-time streaming and analytics), Twitter (high-throughput feed timelines with eventual consistency), Amazon (event-driven microservices at massive scale). HighScalability.com and InfoQ often have articles on these. For example, Netflix’s use of Kafka for real-time data pipelines or how Twitter denormalizes data to serve tweets faster. Pick one system you’re interested in and do a mini “architecture teardown”: identify the core components and the reasoning behind them.
Key Resources (Intermediate)
- Book – Building Microservices by Sam Newman: This book provides practical advice on splitting a monolith, inter-service communication, and the operational facets of microservices. Chapters on integration techniques (synchronous vs messaging) and distributed system pitfalls are directly applicable (The First Law of Software Architecture: Understanding Trade-offs – DEV Community) (The First Law of Software Architecture: Understanding Trade-offs – DEV Community). As you build event-driven services, refer to relevant sections (e.g., how to handle partial failures between services, the need for idempotency in message handling). Newman’s discussion on trade-offs (like the overhead of distributed systems vs the agility benefits) will sharpen your reasoning.
- Book – Designing Event-Driven Systems (O’Reilly) by Ben Stopford: A deep dive into event-driven architecture patterns and best practices. This resource can guide your event pipeline project. Focus on chapters about event brokers, event schemas, and patterns like Event Sourcing and CQRS – they provide insight into when to use which pattern. Stopford also emphasizes the importance of thinking in events and designing around eventual consistency.
- Apache Kafka Documentation and Kafka Summit Talks: Kafka is a gold standard for event streaming. Reading the Kafka intro documentation will teach you why Kafka is built the way it is (sequential disk writes for throughput, partitioning for scalability, acknowledge mechanisms for durability). If possible, watch a Kafka Summit talk like “Kafka Internals 101” or “How LinkedIn uses Kafka” – these often discuss trade-offs Kafka made (e.g., choosing availability over strict consistency, requiring consumers to handle duplicates, etc.). Even if you used RabbitMQ in your project, understanding Kafka’s design will prepare you for larger-scale event systems.
- “The Log: What every software engineer should know about real-time data” by Jay Kreps – an influential blog post that explains the role of log-based messaging (like Kafka) in integrating real-time systems. This will cement your understanding of why event logs are powerful.
- System Design Interview resources (selected): Continue to use system design interview questions as learning exercises, but now focus on ones involving data streams or high throughput. For instance, look at solutions for “Design a news feed” or “Design YouTube” – note how they involve caches, eventual consistency, and often an async component for fan-out. Sites like Exponent or Educative have such case studies. They can validate that your approach aligns with known best practices.
- Community & OSS:
- Kafka or RabbitMQ GitHub: Skim the wiki or design docs of these projects. For Kafka, look at the “Kafka Distributed Systems Design” section in its wiki. For RabbitMQ, read about how it implements reliable delivery. This is less formal than a book but gives insight into real-world engineering trade-offs (e.g., RabbitMQ uses ACKs and persisted queues to guarantee delivery but at cost of throughput, whereas Kafka’s design maximizes throughput with sequential writes and lets consumers handle duplicates).
- Open-Source Example Systems: There are open-source clones or simplifications of big systems (e.g., “mini-Redis” or “mini-HDFS” projects on GitHub, or a clone of Twitter’s timeline). Contributing or even studying their code can be eye-opening. For example, NATS.io (an open-source messaging system) is simpler than Kafka; you could read its documentation or try running it as an alternative to RabbitMQ to compare design philosophies (NATS is built for simplicity and speed with a trade-off in message durability).
- Videos & Courses:
- “Scalable System Design” lectures – Many universities and conferences have intermediate-level talks on building at scale. Search for topics like “Building Real-Time Systems at Scale” (e.g., a GCP online talk on building real-time dashboards) or “Designing Microservices – Martin Fowler” (Fowler has talks on event-driven microservices).
- YouTube Channels (Gaurav Sen / System Design Interview): Channels like Gaurav Sen’s often break down system design problems and solutions visually. Watch episodes related to what you’re building (e.g., “Designing a chat service”, “Designing Uber”, “Message Queue design”). They provide frameworks for approaching such problems and mention the trade-offs and alternatives.
- Conference talks on EDA: For instance, “Turning the database inside-out” by Martin Kleppmann (about moving from batch processing to streaming) – great for understanding why event-driven architectures are adopted and the trade-offs of going real-time versus batch.
Optional Deep Dives (Intermediate):
- CAP and Consistency Models: Read “PACELC theorem” (extends CAP by considering latency vs consistency trade-off when no partition) and Jepsen test reports for databases. This is advanced consistency theory but will enlighten you on how different systems choose trade-offs (e.g., why Cassandra is AP (available, partition-tolerant) and gives up immediate consistency).
- Protocols: Dive deeper into gRPC and Protocol Buffers if you used them. Understand how they achieve efficiency. Perhaps implement a small gRPC service to compare with a JSON HTTP service (optional experiment to measure CPU and network differences).
- Security & Auth: While not the main focus, by this stage it’s good to consider how to design authentication and authorization in distributed systems. Read up on OAuth2 for APIs, token-based auth for WebSockets, and securing message brokers. The trade-off here is often security vs performance vs complexity (e.g., OAuth token introspection adds overhead per call). Ensure you at least conceptualize how your designs secure data and handle multi-tenant scenarios.
Validate Your Understanding
As you conclude Phase 2, you should have tangible systems built and a wealth of new knowledge. Validate this phase with more challenging tests:
- System Design Presentation: Pick one of your projects (say the Real-Time Chat or the Event Pipeline) and pretend you are presenting its architecture to a panel (or actually do so with colleagues). Cover the requirements, your design (draw a diagram with components), and critically, the decisions and trade-offs. For example: “We use Redis Pub/Sub for message distribution to keep it simple, which trades off persistence (messages may be lost if a server goes down). For our use-case (chat), we decided this was acceptable to favor low latency. If we needed guaranteed delivery, we could introduce Kafka but with more complexity and delay.” Such articulation is the clearest sign of mastery of what you built.
- Mock Interview (Complex scenario): Try designing a system you haven’t built but now have the knowledge to tackle, like “Design Instagram” or “Design an Uber-like system”. You’ll find you can reason about real-time updates (feeds or driver locations), high throughput APIs (posting content or matching riders), and asynchronous processing (sending notifications, updating search indices) much more concretely now. Write down your solution outline and ensure you mention how real-time events flow, how you’d scale to millions of users, etc. Compare with others’ solutions or get feedback from a peer – specifically on whether you considered edge cases and trade-offs sufficiently.
- Contribute & Discuss: Engage in an online forum or community. For instance, answer a question on Stack Overflow or Reddit about system design (many ask “How do I scale X?”). Or join a “system design discussion” group (there are Slack/Discord communities for system architects). Explaining your reasoning to strangers is a great test – if you can convincingly argue for a design approach and address their counterpoints, you’re internalizing the principles well.
- Review Real Designs: Take a moment to reflect on your own company’s architecture (if applicable). Map it out and see if you can identify where it uses real-time or event-driven techniques. Perhaps even volunteer to review a design document at work. By seeing theory applied in production, and possibly finding areas for improvement, you validate your new perspective.
- Benchmarking Experiment: As a mini-test, perform a controlled experiment on one of your systems. For example, intentionally remove the cache from your high-throughput API and see the performance drop, or introduce a small delay in your event consumer to simulate a slow consumer and watch the queue backlog grow. This hands-on validation cements why those components are necessary.
By now, you have designed and scaled systems that are not trivial. You’ve likely run into new challenges (maybe debugging concurrency issues or ensuring message order). Celebrate this progress – you’re much closer to “system design mastery” and ready to tackle even more advanced topics.
Phase 3: Advanced – Scaling, Distributed Patterns, and Optimization
In the Advanced phase, you’ll tackle the high end of scale and complexity. This is where system design gets truly challenging and interesting – dealing with distributed system pitfalls, optimizing for high performance, and ensuring reliability under heavy load or failure conditions. The focus will be on advanced architecture patterns (like event sourcing, sagas), large-scale data processing, and fine-tuning systems for efficiency. You will also simulate real-world conditions like failures and see how to design for resilience. By the end of this phase, you should be capable of designing systems for 10M+ users or extremely high throughput (hundreds of thousands of ops/sec), and reasoning about any trade-off (performance, cost, complexity, consistency, etc.) at an expert level.
Goals
- Master advanced architecture patterns: Learn and apply patterns such as Event Sourcing, CQRS, Saga pattern for distributed transactions, circuit breakers, and more. Understand not just how to use them, but why (the problem each solves and the trade-offs incurred, e.g. complexity vs consistency) (Roadmap to Backend Programming Master: Real-Time Data | by Lagu | Medium) (Event-Driven Architecture Roadmap | Deepak Bhardwaj | 13 comments).
- Achieve high performance and throughput: Be comfortable optimizing systems – from efficient algorithms and data structures in code to system-level tweaks (threading model, non-blocking IO, batching, etc.). Aim to design systems that effectively use hardware and network (e.g., can saturate network bandwidth or fully utilize CPU cores without bottlenecks).
- Design for fault tolerance and reliability: Embrace the idea that failure is inevitable. Learn to design systems that gracefully handle failures: data center outages, server crashes, network partitions. Techniques include redundancy, failover, retries with backoff, idempotent operations, and chaos testing. By the end, given a design, you should be able to point out its single points of failure and how to mitigate them.
- Real-world scale data processing: Gain experience with large-scale data pipelines or streaming (processing millions of events) and with specialized data stores (time-series DBs, columnar analytics DBs, etc.). The goal is to handle big data in real-time, bridging the gap between online request processing and offline analytics with approaches like Lambda/Kappa architecture.
- Leadership in design: Start thinking like a software architect or tech lead. This means evaluating designs in terms of business requirements and constraints (throughput needs, development cost, operational cost, team expertise) and guiding others in making trade-off decisions. Essentially, you should be able to not only come up with a design, but also defend it or critique someone else’s design rationally.
- Capstone readiness: Prepare to undertake a “capstone” project that mimics designing a production system end-to-end with a high level of rigor (design docs, code, testing, deployment). This will solidify everything learned and serve as proof of your mastery.
Core Topics to Cover
- Event Sourcing & CQRS (Advanced): Dive deep into Event Sourcing: storing state as a log of events rather than current state. Understand its benefits (auditability, ability to rebuild state, temporal queries) and challenges (need to replay events, evolving event schemas). Implementing event sourcing usually goes hand-in-hand with CQRS, where you have separate read models. Grasp how a system might accept commands (which result in events appended to a log) and then asynchronously update various read databases. Trade-offs: Event sourcing provides ultimate flexibility and decoupling of write/read, but introduces eventual consistency and complexity in managing event schemas. Many real systems (like banking ledgers, or player state in gaming) use this pattern for high integrity and scale (Roadmap to Backend Programming Master: Real-Time Data | by Lagu | Medium) (Roadmap to Backend Programming Master: Real-Time Data | by Lagu | Medium).
- Distributed Transactions & Saga Pattern: In a microservices context, a single user action may involve multiple services (e.g., placing an order involves payment, inventory, and shipping services). Two-phase commit (2PC) is one way to do a distributed transaction, but it’s often avoided due to blocking and complexity. Instead, the Saga pattern is used: a sequence of local transactions with compensating actions on failure. Learn the difference between choreography vs orchestration: choreography (each service listens for events and does next step) vs orchestration (a central coordinator tells each step). Trade-offs: Sagas embrace eventual consistency (each step eventually completes or compensates) and are complex to design (need to handle many failure modes), but they remove the need for a distributed lock on multiple services (Event-Driven Architecture Roadmap | Deepak Bhardwaj | 13 comments). Practice designing a saga for a sample process (like travel booking with flight, hotel, car – if one fails, compensate others).
- Scalability Patterns: Advanced patterns like Sharding strategies (beyond simple hashing – e.g., consistent hashing as used in distributed caches and systems like Cassandra; or sharding by user geography vs random sharding and the trade-offs in balancing load vs locality), Bulkheading (isolating parts of the system so a failure in one doesn’t cascade – e.g., separate thread pools for different tasks), and Backpressure & Throttling (designing the system to handle overload by shedding load gracefully). Understand concepts like queue length as a signal of backpressure and how to propagate backpressure in event pipelines (e.g., Kafka can use consumer lag metrics to slow producers).
- Performance Tuning and Profiling: Learn to identify bottlenecks in a system. This could mean using profiling tools (CPU profiler, flame graphs) to optimize code, understanding how garbage collection (GC) pauses can affect latency (Java’s G1 vs ZGC, or Python’s GIL issues), and using techniques like batching (process 100 requests at a time instead of 1 by 1 to improve throughput) and vectorized operations (especially for data processing, e.g., using numpy/pandas in Python or SIMD operations in C++). Explore how high-performance systems use lock-free data structures or memory pooling to avoid GC overhead (e.g., LMAX Disruptor pattern). Not all of this will be directly implemented, but be aware of what’s possible. Goal: You should be able to reason, for instance, “If I need this service to handle 100k req/sec, I may need to use language X or technique Y because language Z’s runtime would struggle with GC at that rate; or I will need to horizontally scale to N instances and put a load balancer.” Basically, tie performance characteristics to design choices.
- Advanced Storage Systems: Expand your knowledge of storage beyond basic SQL/NoSQL: look at time-series databases (like InfluxDB or TimescaleDB) for metrics, distributed search engines (Elasticsearch) for text queries, graph databases for relationship-heavy data, and columnar stores (like Cassandra or BigQuery) for analytics. Each of these is designed with a specific workload in mind. For instance, Cassandra sacrifices ACID transactions to achieve horizontal scale and fast writes (often chosen for event logging at scale) – a conscious trade-off (15 System design tradeoffs for Software Developer Interviews – DEV Community) (15 System design tradeoffs for Software Developer Interviews – DEV Community). By learning these, you can choose the right tool for different parts of a system (polyglot persistence).
- Multi-Region and Geo-Distribution: Designing systems that work across data centers/regions introduces new challenges: higher latencies, need for geo-replication, dealing with network partitions regularly. Learn techniques like leader-follower replication across regions, conflict-free replicated data types (CRDTs) for state synchronization without central coordination (advanced but interesting for collaborative real-time systems), and traffic routing strategies (DNS load balancing, anycast, active-active vs active-passive failover). Understand concepts of consensus (Paxos/Raft algorithms) at a high level – these underpin distributed databases and coordination services (like etcd/Consul used for service discovery and config). You might not implement Raft from scratch (though it’s a good exercise if inclined), but knowing how it works will let you reason about when you can get a strongly consistent view (with a cost to availability).
- Reliability Engineering: Borrowing from Site Reliability Engineering practices: learn about SLOs/SLAs (e.g., “99.99% uptime” means < ~52 min downtime/year), error budgets (how much unreliability can be tolerated), and chaos engineering (actively testing failures). Tools like Netflix’s Chaos Monkey randomly kill instances – understand why this is done (to ensure the system is robust). Explore patterns like circuit breakers (e.g., Netflix Hystrix library concept – to stop cascading failures by cutting off calls to a failing service) and fallbacks (degraded modes of operation if a component is down). By internalizing these, you’ll design systems with resilience in mind, not just happy-path performance.
- Cost Considerations: At a large scale, cost becomes a design parameter. Learn to evaluate how choices affect the cost – e.g., using managed cloud services vs self-hosting, optimizing for better hardware utilization vs over-provisioning. Understand the basics of cloud pricing (CPU hour, GB-month, network egress costs) to make architecture decisions that are not just technically sound but also cost-efficient. For example, a design that requires doubling data storage might be fine technically but double costs – maybe a more complex but storage-efficient design is preferred if cost is a concern. Mastery includes aligning design with business constraints.
Projects to Build (Advanced/Capstone)
These projects are meant to push your limits and integrate everything. They can be seen as capstones – you might choose one large capstone or a couple of smaller ones. The key is to simulate production-grade system design: include design docs, code, tests, and even deployment scripts if possible. Importantly, incorporate resilience and scale features and test them.
- 7. Capstone Project – End-to-End Scalable Application: Design a full system from scratch as if you were going to launch a startup product to millions of users. Pick a domain you like (for example: a real-time collaboration platform, a ride-sharing service, a live analytics dashboard for IoT, or a massive multiplayer game backend). The system should involve multiple services and demonstrate real-time/event-driven aspects. For instance, if you choose a ride-sharing service (Uber-like): You’ll have services for passenger requests, driver location updates (coming in as a real-time stream), dispatch logic (matching algorithm), a notification service to alert drivers/passengers, etc. Outline the architecture in a design document first – identify services, databases, communication patterns, and justify choices. Then implement a simplified version: you don’t need all Uber features, maybe just simulate drivers and riders on a grid and match them. Use event-driven updates for driver locations (Kafka stream of GPS coords) and a processing service that assigns drivers to riders (this could use a simplified algorithm but structured as a saga if payment involved). Ensure to incorporate advanced patterns: e.g., use saga for the workflow of “assign driver -> if payment fails or driver no-show, cancel and notify” with compensating actions. Use event sourcing for something like ride status changes – log events (“ride requested”, “driver assigned”, “ride started”, “ride completed”) and have a projector that builds a current view for customers. While implementing, simulate scale where possible: maybe use dummy data to simulate 10k drivers. This capstone will likely be the most extensive thing you build – treat it like a real system: version your APIs, include monitoring (e.g., expose metrics from your services), handle at least one failure scenario (what if a service crashes mid-process? do you retry from event log?). After building, do a capacity estimate: e.g., “With the current design, I can handle X requests/sec before the dispatch service becomes CPU-bound – I would scale by adding Y more instances or partitioning drivers by region.” Even if approximate, this thinking is key at mastery level. Finally, document the trade-offs you made: maybe you chose eventual consistency in dispatch for simplicity – write that down and reason about its impact (e.g., a slightly stale driver location might match a not-quite-closest driver, which Uber accepts for scalability).
- 8. Big Data Streaming Pipeline: Build a system capable of ingesting and processing a large stream of data in real-time (or near real-time). For example, create a real-time analytics pipeline for website clicks or sensor data. Use Apache Kafka as the data backbone. Produce a high volume of events (you can write a generator that pumps, say, 100k events per minute into Kafka). Then have multiple processing components: e.g., one that aggregates stats (counts events per type/minute using a sliding window – you could use Kafka Streams or Apache Flink for this), and another that detects anomalies (e.g., sudden spike in events) and issues alerts. Use a data store optimized for time-series (maybe InfluxDB or even Kafka itself with a retention policy for the raw log, plus a separate store for aggregates). The challenge here is handling volume: tune Kafka partitions and consumers to scale horizontally. Experiment with backpressure: e.g., deliberately slow down a consumer and watch Kafka’s lag increase; then add another consumer to the group to catch up, demonstrating horizontal scaling. This project teaches you about stream processing frameworks and their internal trade-offs (Flink provides exactly-once processing with two-phase commit mechanism – see how that adds latency; Kafka Streams provides “at least once” by default which is faster but might double-count on failure). It also forces you to think about event time vs processing time, out-of-order events, etc., which are advanced considerations in event-driven systems. Validate by seeing if your pipeline can keep up with the input rate, and what the end-to-end lag is. If it starts to fall behind, identify the bottleneck (CPU, network, etc.) and optimize or scale that part.
- 9. Fault Tolerance Drill on an Existing Project: Take one of your major systems (perhaps your Phase 2 chat or the Phase 3 capstone) and subject it to chaos testing. Simulate servers going down: e.g., if you have 3 instances of a service behind a load balancer, kill one while a test load is running – does the system continue working? If using Kafka, kill the Kafka broker leader and see if the consumer resumes (you’ll learn about Kafka leader election). Induce a network partition scenario if possible (e.g., make the DB temporarily unreachable). This exercise will show you how resilient your design truly is. It will likely reveal any single points of failure you missed. To improve, implement what real systems do: e.g., add a retry with exponential backoff for a client calling a service that might be down; implement a circuit breaker – if a service is unresponsive for 30 seconds, make the callers stop calling it for a bit and use a fallback (perhaps return cached data or a graceful message). By actually coding these resilience features, you learn the mechanics of reliability. After fixes, repeat the chaos experiments until your system can handle them. This is the closest you can get to simulating a production outage scenario as practice. Document the outcome: “When the primary DB was down, the read replica served reads (stale by up to 5 seconds) – the system continued in read-only mode. Once primary came back, writes resumed. This trade-off (availability over consistency for a brief period) is acceptable to meet our uptime requirement.” This level of insight is what makes one a master architect.
These projects, especially the capstone, are substantial. It’s okay if they take longer than the nominal weeks – the learning is what matters. You can also team up with others or use open-source components to focus on design and integration rather than reinventing everything. The end result should be something you could present to a senior engineering panel or use as a case study in a system design interview for a top company.
Key Resources (Advanced)
- Book – Site Reliability Engineering (Google SRE book): This is an excellent resource on running systems at scale. Focus on chapters like “Reliable Product Launches”, “Monitoring Distributed Systems”, and “Managing Overload”. The SRE perspective will influence how you design (e.g., aim for automated recovery, and embrace simplicity where possible). There’s also a chapter on “Distributed consensus” which demystifies things like Paxos in practical terms. The SRE book is full of real anecdotes of failures – learning from those will inform your designs (for example, the importance of exponential backoff in network calls to avoid thundering herd issues).
- Book – Release It! by Michael Nygard: This book is all about designing and releasing systems that withstand the realities of production. It catalogs failure patterns and stability patterns (like circuit breakers, bulkheads, and steady-state vs burst handling). It’s very practical and written from experience. Read about the common failure modes (e.g., memory leaks, queue overflows) and how to mitigate them in design. Nygard’s patterns will directly help in your fault tolerance drill project.
- Book – Designing Data-Intensive Applications (continued): Finish the latter chapters of DDIA now – especially the ones on Stream Processing and Batch Processing. Kleppmann discusses Lambda Architecture, the trade-offs between batch and streaming (latency vs completeness) (10 System Design Tradeoffs You Cannot Ignore – ByteByteGo) (10 System Design Tradeoffs You Cannot Ignore – ByteByteGo), and real-world systems like Hadoop, Storm, etc. This will reinforce your big data pipeline project. Also, the chapter on Distributed Systems in DDIA covers consistency, consensus and anti-entropy mechanisms in databases – important theory backing your advanced designs.
- Research Papers: Challenge yourself to read a few seminal engineering research papers. Good ones that are very relevant:
- Google’s Spanner paper: Describes a globally distributed SQL database that achieves external consistency with GPS and atomic clocks. It’s heavy, but scan for how they manage to get consistency across data centers (trueTime API). It shows an extreme of trade-off: they added atomic clocks hardware to minimize uncertainty in distributed timestamps – not your everyday solution, but enlightening on pushing boundaries.
- Amazon’s Dynamo paper: This paper inspired many NoSQL systems (Cassandra, Riak). It explains the decisions for an AP (Available/Partition-tolerant) system: allowing eventual consistency and conflicts (like divergent versions of data) in exchange for always being available (15 System design tradeoffs for Software Developer Interviews – DEV Community). It introduces vector clocks for conflict resolution. It’s a great illustration of many concepts you’ve learned: hashing (for partitioning), eventual consistency, hinted handoff, etc.
- “Out of the Tar Pit”: An essay discussing simplicity vs complexity in software design, making the case that a lot of system complexity arises from state and how functional programming (immutable events) can simplify. This resonates with event sourcing and CQRS – it provides a philosophical rationale for those patterns (reducing mutable state).
- Any recent case study from ACM Queue or Communications of the ACM: e.g., articles titled “X at Scale” (like “Dropbox at Scale” or “Facebook TAO: the power of the graph”). These often contain gold nuggets of trade-off analysis by engineers who built giant systems.
- Advanced Topics Learning:
- Chaos Engineering Resources: The principles of chaos engineering (e.g., the Chaos Monkey guide by Netflix, or newer blog posts on chaos tests in Kubernetes) will give you ideas for your fault tolerance tests.
- Performance Tuning: If your interests lean that way, resources like Brendan Gregg’s “Systems Performance” or Martin Thompson’s blogs (Mechanical Sympathy) can teach you about how to squeeze out performance by understanding OS and hardware. While you might not need this detail for system design interviews, it is incredibly useful when making high throughput systems in real life (e.g., understanding context switch costs, NUMA memory effects, etc.).
- Distributed Systems Courses: At this stage, you could even take an online course like MIT 6.824 (Distributed Systems) or Stanford CS244b (Distributed Systems) to formalize your understanding. These involve coding labs (like implementing Raft, building a small KV store) – time-consuming but the best way to truly grok consensus and fault tolerance. Consider this if you want to solidify theory with practice at a deep level.
- Case Studies & Post-Mortems:
- Netflix, Google, and Amazon Blog Posts: These companies often share deep dives. For example, Google Cloud’s blog might discuss their internal spanning tree for Pub/Sub, or Netflix tech blog has posts like “Evolution of our Edge Proxy” (how they designed Zuul). These are advanced but seeing the decision process at these firms is educational.
- Post-Mortems: Reading failure post-mortems (just Google “post-mortem outage cloud service”) is extremely enlightening. You learn what went wrong and what design decisions could have prevented it. For instance, the AWS DynamoDB outage post-mortem (2015) highlighted issues with overwhelming recovery tasks – they throttled themselves while recovering from failure, which taught the industry to design graceful degradation rather than trying full recovery under pressure. Collect a few relevant to your projects and see how advanced designs handle failure.
- Community & Contribution:
- Open Source Contribution: By now, you have significant skills – contributing to a major open source project is a great test and learning experience. For example, try to contribute to Apache Kafka, or a smaller but related project like Apache Pulsar (another distributed pub-sub system). Even fixing a minor bug or improving docs will force you to read and understand parts of the system’s design. The code review feedback from maintainers (if any) can also be educational.
- Architecture forums or blogging: Start answering questions on high-level forums (Stack Exchange Software Engineering, Reddit r/architecture). Even consider writing a blog series about what you’ve built/learned (e.g., “Designing a Scalable Chat System – Lessons Learned”). Teaching is the final step of learning – articulating your advanced knowledge for others cements it for you and highlights any areas you need to clarify for yourself.
Optional Specializations (Advanced): By this point, you might find a particular area especially interesting. You can opt to deep dive into a specialization:
- Real-Time Machine Learning Systems: If ML is of interest, learn about feature stores, model serving at scale, concept drift – how to design systems that serve ML predictions in real-time (e.g., recommendation engines that update with events). This combines streaming and high throughput with new challenges (ensuring model accuracy, etc.).
- Blockchain and Decentralized Systems: A very different take on distributed systems – consensus in hostile environments, eventual consistency writ large. Studying how blockchain networks achieve reliability (PoW, PoS algorithms) can broaden your thinking on consensus and trade-offs (security vs throughput, decentralization vs efficiency).
- Edge Computing and IoT: Systems where data is processed close to where it is produced (for latency or bandwidth reasons). This has unique design aspects like intermittent connectivity, constraint device resources, etc.
- Enterprise Integration Patterns: If you expect to work on integrating many enterprise systems, look into patterns from the EIP book (by Hohpe/Woolf) like messaging gateways, content-based routing, etc., which can be seen as an advanced form of event-driven design in enterprise contexts.
Validate Your Mastery
In this phase, validation is about real-world readiness and the ability to handle ambiguity and novel problems:
- System Design Mock with a Twist: Have a friend give you an unknown, crazy scenario to design – something not common or that pushes limits, like “Design a system to provide real-time earthquake alerts worldwide” or “Design a global multiplayer chess platform for 100 million users with move broadcasts under 100ms.” These kind of problems require you to think of everything – from real-time requirements to global distribution and fault tolerance. The goal is to see if you can quickly break down the problem, list assumptions, and outline a solution using your repertoire of techniques. After you propose a design, critique it yourself: where are the risks? what could fail? what are the alternative approaches? If you can conduct this level of self-review, you’ve achieved a mastery mindset.
- Formal Design Document: Write a full design document for one of your major projects as if you were going to hand it to a team for implementation. Include sections: Requirements (SLAs, scale, use cases), Proposed Architecture (diagrams, component roles), Data models, Trade-off Discussions (why chosen tech, what alternatives considered), Failure Modes and how handled, Deployment and Scaling plan, Monitoring plan, etc. Use a structure similar to what big companies use for design reviews. This exercise ensures you consider all aspects of a production system. It’s also great practice for communicating your design – a key skill at mastery level. If possible, get a senior engineer or architect to review this document and give feedback.
- Lead a Design Review (simulation): Simulate being the “architect in the room” for a design review. You can do this by taking an existing design (maybe from a blog or a friend’s project) and identifying issues or improvements. For example, review the architecture of an open source project (like examine how WordPress architecture works or a smaller scale system) and write a critique with suggestions. Alternatively, mentor a junior developer in designing something simple, and guide them rather than doing it for them. If you can mentor someone else through a system design, that’s a strong validation of your mastery – you’ll be forced to explain the ‘why’ of choices clearly and adjust the plan based on their questions.
- Performance/load testing at scale: If you have access to resources (or cloud credits), try to push one of your systems to a large scale in a controlled test. For example, deploy your capstone project on cloud instances and use a load generator to simulate 1 million users (there are cloud-based load testing tools that can generate huge loads). Observe how the system behaves in terms of CPU, memory, network. Does it auto-scale? Does it crash? This kind of testing might require $$ for cloud, so if that’s not feasible, mentally work through a capacity plan: e.g., “To handle 1 million concurrent users, I’d need X servers of type Y; the bottleneck would likely be Z – and here’s how I’d alleviate it.” The ability to estimate and plan capacity is a hallmark of experienced architects.
- Job interview prep (if relevant): At this point, you should feel confident to tackle even the toughest system design interviews (like FAANG-level or top-tier companies). It might be worth doing a few mock interviews with industry peers or using interview platforms to ensure you can apply all this knowledge under time pressure. The feedback from these (if any weak areas remain) will highlight final areas to shore up.
Finally, reflect on how far you’ve come – from basic concepts to designing complex, distributed, real-time systems. Mastery is not an endpoint but a continuous journey. However, with this intensive roadmap, you will have achieved a level of skill where you can confidently design and reason about systems of any scale, always mindful of the trade-offs and aligned with real-world constraints.
Below is a summary table of the roadmap phases, highlighting the focus, key topics, deliverables, and estimated duration for each:
Phase (Duration) | Focus & Core Topics | Key Deliverables/Projects | Estimated Time |
---|---|---|---|
Phase 1: Foundation (4 weeks) | – Scalability basics (vertical vs horizontal) – Performance vs throughput (15 System design tradeoffs for Software Developer Interviews – DEV Community), CAP theorem (System Design Roadmap: A Step-by-Step Guide to Mastering System Design – DEV Community), consistency models – SQL vs NoSQL basics (15 System design tradeoffs for Software Developer Interviews – DEV Community); Caching & CDNs (System Design Roadmap: A Step-by-Step Guide to Mastering System Design – DEV Community) – Client-server, stateless vs stateful (15 System design tradeoffs for Software Developer Interviews – DEV Community) – Intro to real-time (WebSockets vs polling) (15 System design tradeoffs for Software Developer Interviews – DEV Community) – Intro to event-driven (queues, pub/sub) | – Real-time Notification Demo: Basic WebSocket broadcaster – Scaled API Demo: Nginx load-balanced app with Redis cache (System Design Roadmap: A Step-by-Step Guide to Mastering System Design – DEV Community) (System Design Roadmap: A Step-by-Step Guide to Mastering System Design – DEV Community) – Async Processing Demo: Queue + worker for background task | ~4 weeks (32 hrs) |
Phase 2: Intermediate (8 weeks) | – Message brokers (Kafka, RabbitMQ) & pub/sub ([Event-Driven Architecture Roadmap | Deepak Bhardwaj | 13 comments](https://www.linkedin.com/posts/mr-deepak-bhardwaj_event-driven-architecture-roadmap-activity-7285626850486231040-Ne_r#:~:text=start%3F%20Here%E2%80%99s%20a%20100,on%3A%20Demonstrate)) – Event-driven patterns (event sourcing vs event notification basics) – Microservices architecture; API Gateway vs direct comm. – Real-time comm: WebSockets, SSE, gRPC streaming (with backpressure) ([Roadmap to Backend Programming Master: Real-Time Data |
Phase 3: Advanced (8–12 weeks) | – Event Sourcing & CQRS (event log + read models) ([Roadmap to Backend Programming Master: Real-Time Data | by Lagu | Medium](https://medium.com/@hanxuyang0826/roadmap-to-backend-programming-master-real-time-data-88f3a3fa8304#:~:text=5)) – Saga pattern for distributed transactions ([Event-Driven Architecture Roadmap |
Phase 4: Mastery (12+ weeks, ongoing) | – Leadership in architecture: reviewing and guiding designs – Cutting-edge topics as needed (security at scale, new tech paradigms) – Cost vs performance trade-offs on large systems – Continuous learning (staying updated with tech, papers) – Possibly specialization (ML systems, blockchain, etc.) – Polishing communication (writing design docs, giving talks) | – Design Docs & Reviews: Write formal design docs and conduct design reviews for complex systems – Open Source Contribution: to a relevant project (e.g., Kafka, etcd) to deepen practical knowledge – Public Sharing: Blog or talk about an advanced system design topic, demonstrating thought leadership – Mock Interviews & Team Mentoring: Regularly challenge yourself with new design problems and mentor others (for reinforcement) | ~12 weeks (96 hrs) and beyond (continuous) |
Throughout each phase, remember to focus on the reasoning behind decisions. Mastery isn’t just about knowing patterns or tools, but understanding why and when to use them. By following this roadmap and actively engaging with projects and case studies, you will cultivate the mindset and skills of a seasoned system architect, capable of designing robust, scalable systems and articulating the trade-offs of every decision (15 System design tradeoffs for Software Developer Interviews – DEV Community) (The First Law of Software Architecture: Understanding Trade-offs – DEV Community). Good luck on your journey to deep system design mastery!
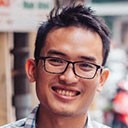
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.