Table of Contents
Variable initialization is an important concept in Java and developers need to understand this topic thoroughly.
In this post, we are going to dive deep into variable initialization in Java 17. At the end of the posts, there are some quizzes to help you understand this topic further.
What is variable initialization in Java?
Before using a variable, you need to give it a value. The act of giving a variable a value is called variable initialization.
In some cases, variable initializations are done automatically. In other cases, the developers need to explicitly give values to variables.
Class, instance, and local variables
Local variables are the ones defined in methods
Class variables are the static variables defined in class
The rest are instance variables
Let’s take a look at a code snippet of such variables
class Cat { private static int totalCat = 0; //class variable private String name; //instance variable public void introduce() { var currentTime = System.currentTimeMillis(); //local variable System.out.println("my name is " + name + " and now is " + currentTime); } }
Automatic variable initialization
Let’s consider the following code snippet
public class Dog { private String name; private float age; private Object behavior; private boolean isOld; private int furCount; public Dog() { System.out.printf("I am a dog with name %s, age %s, behavior %s, isOld %s, fur count %s", name, age, behavior, isOld, furCount ); } }
Let’s also create a new Dog instance
public static void main(String [] args) { var d = new Dog(); }
Here is the output
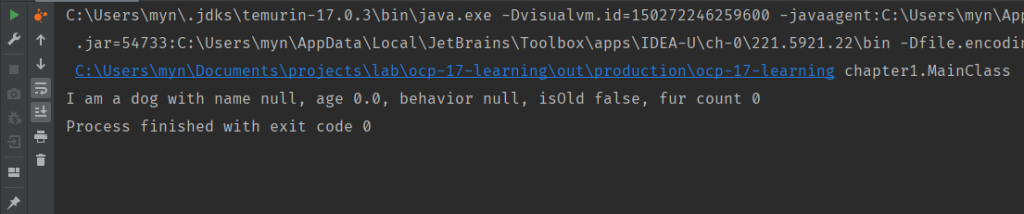
As you can see, all the variables of the class Dog were given their default values depending on their types.
The rules for variable initialization in Java in this: local variables need to be initialized by developers before they can be used. Class and instance variables are given default values if they are not explicitly initialized.
Initialize local variables
Let’s take a look at local variable initialization
public void reportTime() { var currentTime = System.currentTimeMillis(); //local variable String name2; System.out.println("now is " + currentTime); }
In this code snippet, you can see we have two local variables currentTime
and name2
. Do you think this code compiles?
Yes, it does.
The rule of variable initialization is a local variable must be initialized *before it is used*. In this case, the variable name2
is not used so it doesn’t need to be initialized.
Variable initialization using var
From java 11, there is a var
keyword that let you define variables without specifying their types. It’s a method called type inference
.
When using var
, you need to specify the value for the variable because, without the value, the compiler doesn’t know how to infer the type for that variable.
This code will not compile:
public void reportTime() { var currentTime = System.currentTimeMillis(); //local variable var name2; //-> compiler complains System.out.println("now is " + currentTime); }
The final keyword
You can use the final keyword to define a local variable, class variable, or instance variable.
When using the final keyword on class and instance variables, you need to give them values explicitly.
This code will not compile:
class Cat { private final static int totalCat; // need to assign value private final String name; // need to assign value public Cat () { } }
For a class variable, you need to assign a value when declaring it or initialize it in a static block.
For instance variable, you can either assign a value when you declare or in a constructor.
This code snippet compiles just fine:
class Cat { private final static int totalCat = 10; private final String name; private final String breed = "Some cat breed"; public Cat () { name = "James"; } }
This code is also OK:
class Cat { private final static int totalCat; static { totalCat = 0; } public Cat() { } }
One important thing with final instance variables is that if you have multiple constructors, you need to instantiate them in every constructor (if you don’t initialize them when declaring them).
This code will not compile:
class Cat { private final static int totalCat = 10; private final String name; private final String breed = "Some cat breed"; public Cat () { name = "James"; } public Cat(int age) { //need to initialize name in this constructor too } public void introduce() { final String greeting; //this is OK } }
Finally, with local variables, if you don’t use the variable, you don’t need to initialize (even when you declare the variable final
)
This code compiles just fine:
public void introduce() { final String greeting; //this is OK }
Conclusion
In this post, we’ve learned about variable initialization in Java. The most important things to remember are:
- Variables need to have a value before they can be used
- Class and instance variables are assigned default values if you don’t give them values explicitly
- You always need to give local variables values before using them.
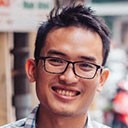
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.