Table of Contents
Using events to pass messages in EJB can be a useful tool to simplify your business logic. Let’s learn how to fire and listen to events in EJB with an example.
Create and listen to simple EJB events
Let’s imagine that we have just opened a store and when someone visits our shop, we will have some fireworks.
So the logic for the event is: When someone comes -> have some fireworks.
Our first step is to create an object named VisitorComesEvent:
package com.datmt.quarkus.events.events; public class VisitorComesEvent { }
It’s a simple object, nothing special.
Now, to fire that event, for example at a rest endpoint, we can do like this:
package com.datmt.quarkus.events; import com.datmt.quarkus.events.events.VisitorComesEvent; import javax.enterprise.event.Event; import javax.inject.Inject; import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.Produces; import javax.ws.rs.core.MediaType; @Path("/store") public class Store { @Inject Event<VisitorComesEvent> visitorComesEvent; @GET @Produces(MediaType.TEXT_PLAIN) public String hello() { visitorComesEvent.fire(new VisitorComesEvent()); return "Welcome to our shop!"; } }
As you can see, when someone visits our shop at /store, the event will be fired.
Now, let’s create a listener to handle the event:
package com.datmt.quarkus.events.events; import javax.enterprise.event.Observes; public class VisitorComesObserver { public void fireWork(@Observes VisitorComesEvent event) { System.out.println("Fireworks!!!!"); } }
It’s another simple object. However, the magic here is the annotation @Observer.
When you open the browser and visit /store, the log will say “Fireworks!!!”.
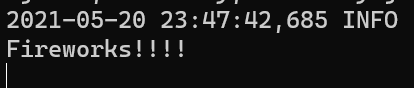
Extending EJB events for specific cases
Now let’s imagine (again) that the store sells toys for kids. When a kid comes to the store, in addition to showing the fireworks, we would like to offer our little customers some candies.
Let’s create another event extend the current event called YoungVisitorComes:
package com.datmt.quarkus.events.events; public class YoungVisitorComesEvent extends VisitorComesEvent{ }
Let’s create another endpoint called /store/young that when someone visits this URL, we will fire YoungVisitorComesEvent instead of VisitorComesEvent:
@GET @Path("/young") @Produces(MediaType.TEXT_PLAIN) public String hello_youngsters() { visitorComesEvent.fire(new YoungVisitorComesEvent()); return "Welcome to our shop, youngster!"; }
The VisitorComesObserver now look like this:
package com.datmt.quarkus.events.events; import javax.enterprise.event.Observes; public class VisitorComesObserver { public void fireWork(@Observes VisitorComesEvent event) { System.out.println("Fireworks!!!!"); } public void candies(@Observes YoungVisitorComesEvent event) { System.out.println("Here are some candies!"); } }
Let’s visit the store as an young customer, we should see Fireworks and also get some candies:
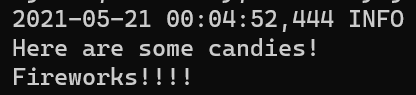
Passing data to the event
Currently, our event objects are quite simple without any properties. Let’s say for example, before showing fireworks and offering candies, we ask the customer’s name so we can print her name on the candies.
Let’s modify YoungVisitorComesEvent like this:
package com.datmt.quarkus.events.events; public class YoungVisitorComesEvent extends VisitorComesEvent{ private String name; public void setName(String name) { this.name = name; } public String getName() { return name; } }
Now, when firing the event, we pass the customer’s name to the event object:
@GET @Path("/young") @Produces(MediaType.TEXT_PLAIN) public String hello_youngsters() { YoungVisitorComesEvent event = new YoungVisitorComesEvent(); event.setName("Jane"); visitorComesEvent.fire(event); return "Welcome to our shop, youngster!"; }
Here, I just hard-coded the name of the customer. However, you should pass the name dynamically in real-life scenario.
Now, in the event handler, we can get the name of the customer like so:
public void candies(@Observes YoungVisitorComesEvent event) { System.out.println("Here are some candies!" + event.getName()); }
Visit the URL again, we should get the messages as expected:
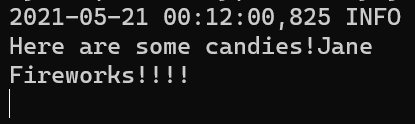
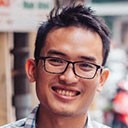
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.