Table of Contents
Often time, when creating web application, you need to set some values in application settings and later, pull such values to display to users.
One of the most prevalent examples is the application’s name and/or application’s version. I’ll show you how to set such values in the app and later, display them in an example endpoint.
Setting custom properties in application.properties
One place you can set custom properties for the application is in application.properties file. For example, I set two properties as follow:
myapp.author=datmt myapp.created_year=2021
Now, let’s create an endpoint and display those values.
Getting custom properties in REST endpoints
Setting properties was easy, getting them is no exception. Let’s see how I get those values in the follow snippets:
@ConfigProperty(name = "myapp.author") String author; @ConfigProperty(name = "myapp.created_year") String createdYear; @GET @Path("info") @Produces(MediaType.TEXT_PLAIN) public String info() { return "App created by " + author + " in the year " + createdYear; }
When accessing the URL in my browser, this is the result:
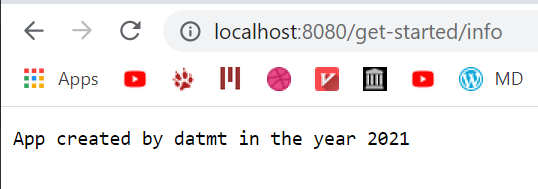
As you can see, the values I set in application.properties file were displayed correctly in the browser.
Setting default fallback value
There are times you want to set a default value for a specific property in case it is not available. You can do that easily with default in @ConfigProperty.
For example, I’ll try to access a property that didn’t set in application.properties file called myapp.language. In @ConfigProperty, besides setting name, I also set a default value:
@ConfigProperty(name = "myapp.language", defaultValue = "French") String language;
In the info method above, I updated the return string to be like so:
@GET @Path("info") @Produces(MediaType.TEXT_PLAIN) public String info() { return "App created by " + author + " in the year " + createdYear + " in " + language; }
When viewing on my browser, I got the language displayed as French since there wasn’t any setting for that property in application.properties file:
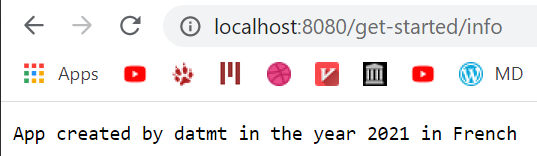
Setting multiple values for property
As you’ve seen above, I set only String to the properties. However, if you want, you can set a list as one property.
myapp.programming_languages=Java,PHP,JavaScript,Go
I created a new endpoint called language-info with the following details:
@GET @Path("language-info") @Produces(MediaType.TEXT_PLAIN) public String languageInfo() { return String.join(",", languages); }
Sure enough, when visiting the endpoint, I got the expected result:
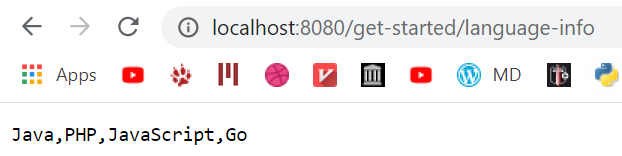
In case you have multiple properties and don’t want to inject each property separately, you can inject Config object:
@Inject Config config;
and get the value using getValue.
String appLanguage = config.getValue("myapp.language", String.class);
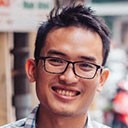
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.