Table of Contents
Overview
As a web developer, you need to interact with APIs/URLs all day. There are many ways you can interact with such resources and get the job done. However, knowing how to CURL can boots your productivity to another level.
In this post, I’m going to show you the most useful CURL commands you can use right now to help you become a more effective developer.
Let’s get started.
Basic CURL commands
Here are some commands to get your feet wet:
Sending GET requests
The default HTTP method curl uses is GET. Thus, to send a GET request to an URL, you only need to call curl with that URL:
curl https://github.com
You will see a lot of text on the screen. This is the HTML content of https://github.com
Save request body to a file
If you want to save the body of the request to a local file, use this:
curl -o github.html https://github.com/
Here, I save the HTML content of https://github.com to a local file named github.html.
Follow redirects
If you make a GET request to http://github.com, you will not see the content you would when visiting it in the browser.
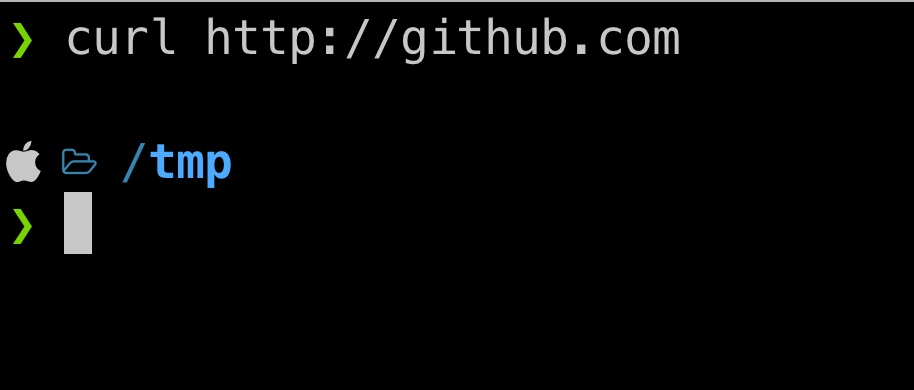
This is because the http endpoint redirects to https.
To tell curl to follow any redirects, you need to use -L
curl -L http://github.com/
You would see the output exactly likes when you query https directly.
More advanced curl queries
Now you know the basic of curl usage, let’s try some slightly advance commands.
Send a request with headers
You can specify the request headers with -H
option. One of the most common use cases of passing a header passing JWT token for authorization.
In such case, you would send this command:
curl -H 'Authorization: Bearer ey....jwt...token...here' https://secure-endpoints.com
If you have multiple headers, simply declare multiple -H
options.
Specify the HTTP method
As mentioned above, the default HTTP method curl uses is GET. If you want to use other methods, specify it with -X
. Remember, there is only one method per request.
# Sending post curl -X POST https://secure-endpoints.com # Sending PUT curl -X PUT https://secure-endpoints.com
Query URL with basic auth
Basic Authen is a simple authentication mechanism you can use to access secured endpoints. To make requests to such endpoints using basic auth, you can use the following command:
# Sending post curl -X POST -u username:password https://secure-endpoints.com
POST JSON data to an endpoint
You can use the following command to send data to and endpoint using POST (PUT/PATCH are similar, you just need to replace the verb).
# Sending post curl -X POST -H "Content-Type: application/json" -d '{"param1": "value1", "param2": "value2"}' https://secure-endpoints.com
To send form data, you can use the following command:
# Sending post curl -X POST -d 'username=johndoe&password=secret' https://secure-endpoints.com/login
Other useful CURL commands
By default, curl output is not verbose. You can add -v
to get more info about the request:
curl -v https://secure-endpoints.com/login
If you are in development and use a self-sign certificate, chances are you will have curl error (60). To bypass SSL check, use -k
option:
curl -k https://secure-endpoints.com/
Conclusion
In this post, I’ve shown you how to use CURL to effectively communicate with URLs and API endpoints. Knowing how to use CURL has proven very beneficial for me in my day-to-day work as a web developer. I believe you would get the same benefits too.
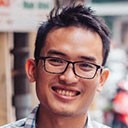
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.