Overview
Recently, I did some overhauls for my JavaFX application. The app has a default Java icon on Windows and Linux but for some reason, on my Mac, the icon is a folder.
I was OK with the default Java icon but no way I can accept it as shown as a folder on Mac.
So, I decided to find a way to set the icon for my app on Mac’s dock.
And it worked!
The code is quite simple though.
How to set the application icon for your JavaFX app
First of all, here is my folder structure:
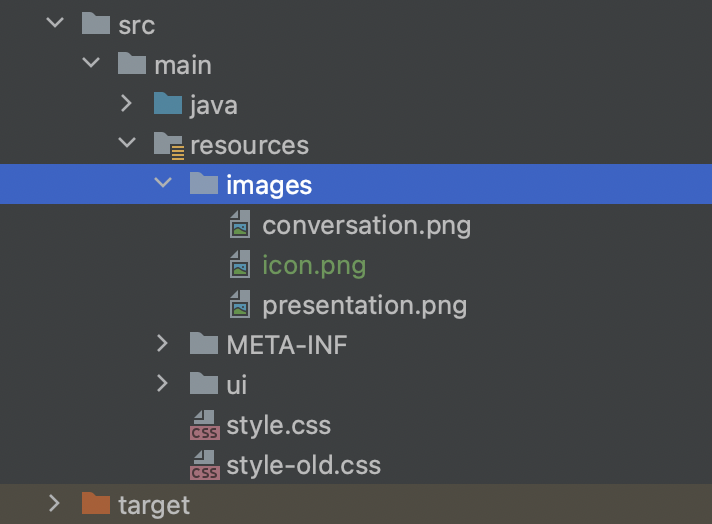
As you can see, the icon.png file is under the images folder.
If you have a different structure, you need to adjust accordingly.
Here is the code snippet that displays your custom application icon:
import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.scene.image.Image; import javafx.stage.Stage; import java.awt.Taskbar; import java.awt.Toolkit; import java.awt.Taskbar.Feature; //.. Other imports public class Main extends Application { @Override public void start(Stage primaryStage) throws Exception { Parent root = FXMLLoader.load(getClass().getResource("/ui/app_ui.fxml")); //Set icon on the application bar var appIcon = new Image("/images/icon.png"); primaryStage.getIcons().add(appIcon); //Set icon on the taskbar/dock if (Taskbar.isTaskbarSupported()) { var taskbar = Taskbar.getTaskbar(); if (taskbar.isSupported(Feature.ICON_IMAGE)) { final Toolkit defaultToolkit = Toolkit.getDefaultToolkit(); var dockIcon = defaultToolkit.getImage(getClass().getResource("/images/icon.png")); taskbar.setIconImage(dockIcon); } } primaryStage.setTitle("Excel Ultimate Search (ver. " + AppInfo.APP_VERSION + ")"); primaryStage.setScene(new Scene(root)); primaryStage.setMaximized(true); primaryStage.show(); } }
There are two parts in the snippet above you need to use:
From line 6 to line 7, this snippet set the icon on the application header. Here is the icon on my app:
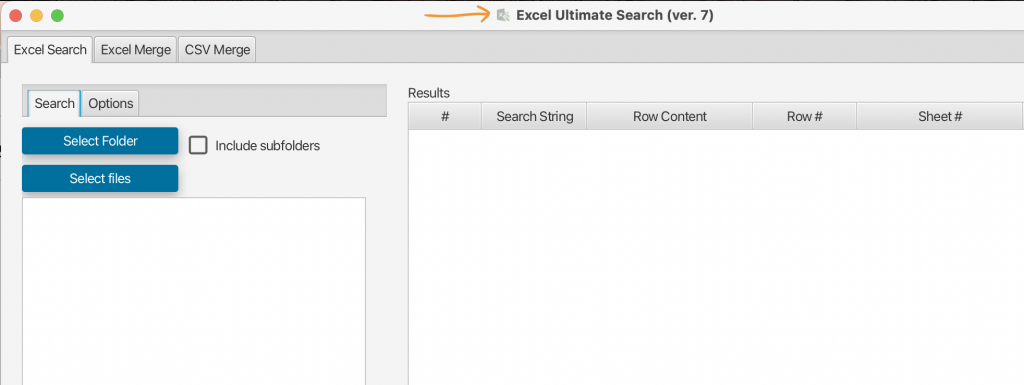
Theses two lines are enough if you use your app on Windows and Linux only.
However, if you also want to support MacOS, you will need 10 to 19.
Line 10 to line 19 do the task of setting the application icon on the MacOS’s dock.
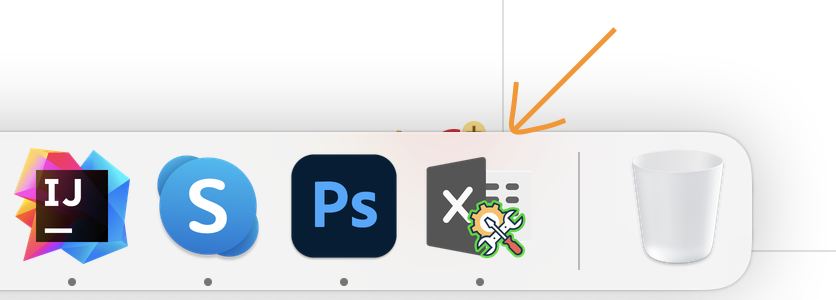
Conclusion
As you can see, it’s quite simple to set the application icon on the title bar and also on the taskbar/dock for your JavaFX application. My development environment uses Java 17 SDK but this should work on other versions too.
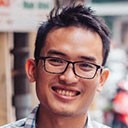
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.
How was Taskbar imported?
I got Taskbar can not be resolved.
Thank you!
import java.awt.Taskbar;
import java.awt.Toolkit;
import java.awt.Taskbar.Feature;
I updated the post with the imports lines.