Overview
With NIO2, you can do things that aren’t available in the old IO. One of such things is you can watch file changes in certain directories.
Let’s see how to do it in action.
Watching file changes in directories using Java
Let’s consider this code snippet:
package com.datmt.self_learning.nio; import java.nio.file.*; import static java.nio.file.StandardWatchEventKinds.*; public class FileWatcher { public static void main(String[] args) throws Exception { // Get the directory to be monitored Path dir = Paths.get("/Users/mac/Downloads"); // Create a WatchService try (WatchService watcher = FileSystems.getDefault().newWatchService()) { // Register the directory with the WatchService for specific events dir.register(watcher, ENTRY_CREATE, ENTRY_DELETE, ENTRY_MODIFY); System.out.println("Watch Service registered for dir: " + dir); // Infinite loop to wait for events while (true) { // Wait for a key to be available WatchKey key = watcher.take(); // Iterate over the events in the key for (WatchEvent<?> event : key.pollEvents()) { WatchEvent.Kind<?> kind = event.kind(); // Context for directory entry event is the file name of entry Path fileName = (Path) event.context(); System.out.println(kind.name() + ": " + fileName); } // Reset the key -- this step is critical to receive further watch events boolean valid = key.reset(); if (!valid) { break; // The watch key is no longer valid } } } } }
This code is quite straightforward. Essentially, it creates a watcher and registers the directory “/Users/mac/Downloads” to that watcher. When there are changes:
- File created
- File updated
- File delete
The watcher will report to the terminal.
Let’s see the watcher in action:
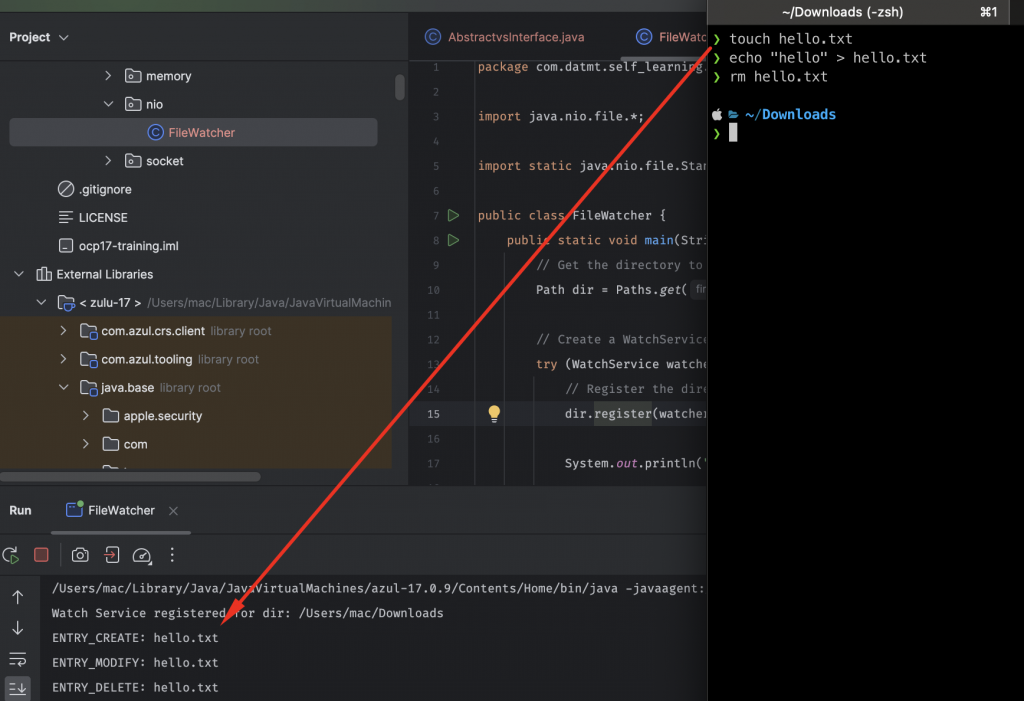
As you can see, the terminal displayed the events as they happened.
Conclusion
In this post, I’ve shown you know to create a watcher for file changes using NIO2 in java.
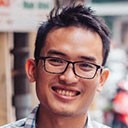
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.