Table of Contents
Overview
In Java, the ==
operator and the equals()
method are both used to compare objects, but they serve different purposes and operate in fundamentally different ways.
Stack, Heap, and References
Before delving into the differences between == and equals, first, you need to understand what are references and how they are stored and used in Java.
References and the Heap
In Java, objects are stored in the heap memory. The heap is a runtime data area from which memory for all class instances and arrays is allocated. It is created at the JVM start-up.
Heap memory for objects is reclaimed by an automatic memory management system known as garbage collection, which frees memory that is no longer reachable by any references.
When a new object is created, for example, Object a = new Object()
, the actual object is placed in the heap, and the variable a
stores a reference to it. This reference is essentially the memory address where the object is stored in the heap. However, the variable a
itself is stored in the stack memory.
Stack Memory and References
Stack memory is used for execution threads and stores primitive variables and references to objects in the heap space. Each thread in a Java application has its own stack that is created when the thread is created. The stack contains frames that hold local variables and references to other objects in the heap.
When a method is called, a new frame is pushed onto the stack for that method’s local variables and references. When the method completes, the frame is popped off the stack. The references stored in the stack memory point to objects in the heap. These references are critical for accessing and manipulating the objects. For instance, when you pass an object to a method, what is passed is the reference to the object. This allows methods to operate on the same object instances stored in the heap.
The == Operator
The ==
operator is used for reference comparison, which means it checks if two object references point to the same memory location. When you use ==
with object references, it will return true
if both references are pointing to the same object instance in the heap, otherwise, it will return false
.
String s1 = "HELLO"; String s2 = "HELLO"; String s3 = new String("HELLO"); System.out.println(s1 == s2); // true, because s1 and s2 refer to the same instance in the string constant pool System.out.println(s1 == s3); // false, because s3 is created in the heap as a new instance
In the above example, s1
and s2
point to the same string literal in the string constant pool, hence s1 == s2
evaluates to true
. However, s3
is created with the new
keyword, which forces the creation of a new string object in the heap, so s1 == s3
evaluates to false
.
The equals() Method
The equals()
method, on the other hand, is intended for content comparison. It checks if two objects are “equal” in terms of their state (the data they hold), not their reference. The behavior of the equals()
method is not defined by the language itself but by the specific implementation within the classes.
By default, the equals()
method in the Object
class compares the references, just like the ==
operator. However, many classes override the equals()
method to provide a more meaningful comparison based on the content of the objects.For example, the String
class overrides the equals()
method to compare the sequence of characters within the two string objects:
String s1 = new String("HELLO"); String s2 = new String("HELLO"); System.out.println(s1.equals(s2)); // true, because the content of both strings is the same
Even though s1
and s2
refer to different objects, s1.equals(s2)
returns true
because the content of both strings is identical.
Key Differences
==
checks for reference equality (whether two references point to the same object).equals()
checks for content equality (whether two objects are logically “equal” based on their content).==
is an operator, whileequals()
is a method that can be overridden.- By default,
equals()
behaves like==
in theObject
class, but it is often overridden in subclasses to provide content-based equality checks.
Conclusion
In summary, use ==
when you want to check if two references are to the same object and use equals()
when you want to check if two objects are equivalent in terms of their data. Always remember that the behavior of equals()
can vary depending on how it’s implemented in the class of the objects you’re comparing.
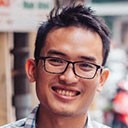
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.