Table of Contents
Data Initialization
Here is the cheatsheet to initialize different Redis data types:
# String SET key value SET name "John Doe" # List RPUSH key element [element ...] RPUSH numbers 1 2 3 4 5 # Set SADD key member [member ...] SADD colors red green blue # Hash HSET key field value [field value ...] HSET user id 1 name "John Doe" email "[email protected]" # Sorted set ZADD key score member [score member ...] ZADD scores 90 "John Doe" 80 "Jane Doe" 95 "Bob Smith"
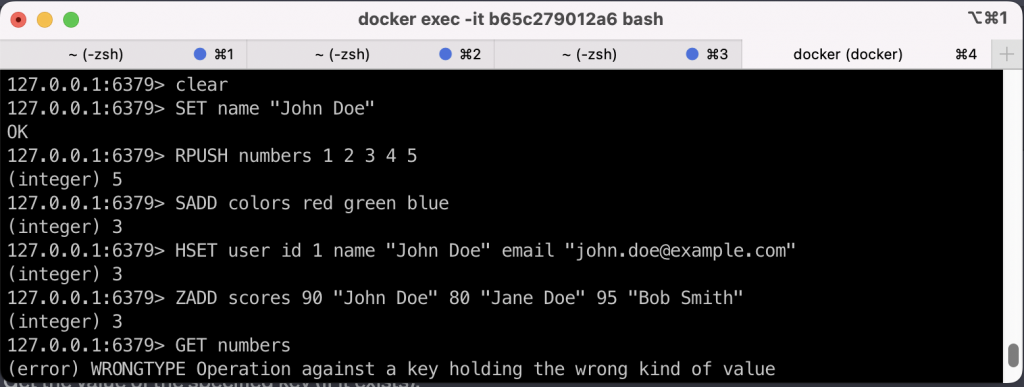
I was able to set the values for different data types. However, when I tried to use GET on a datatypes other than String, I got an error: “(error) WRONGTYPE Operation against a key holding the wrong kind of value”.
Key Commands
SET key value #: set a key to a value GET key #: get the value of a key (string only) DEL key #: delete a key EXPIRE key seconds #: set a timeout on a key TTL key #: get the remaining time to live of a key KEYS pattern #: find all keys matching a pattern SCAN cursor [MATCH pattern] [COUNT count] [TYPE type] #: iterate over keys with a cursor
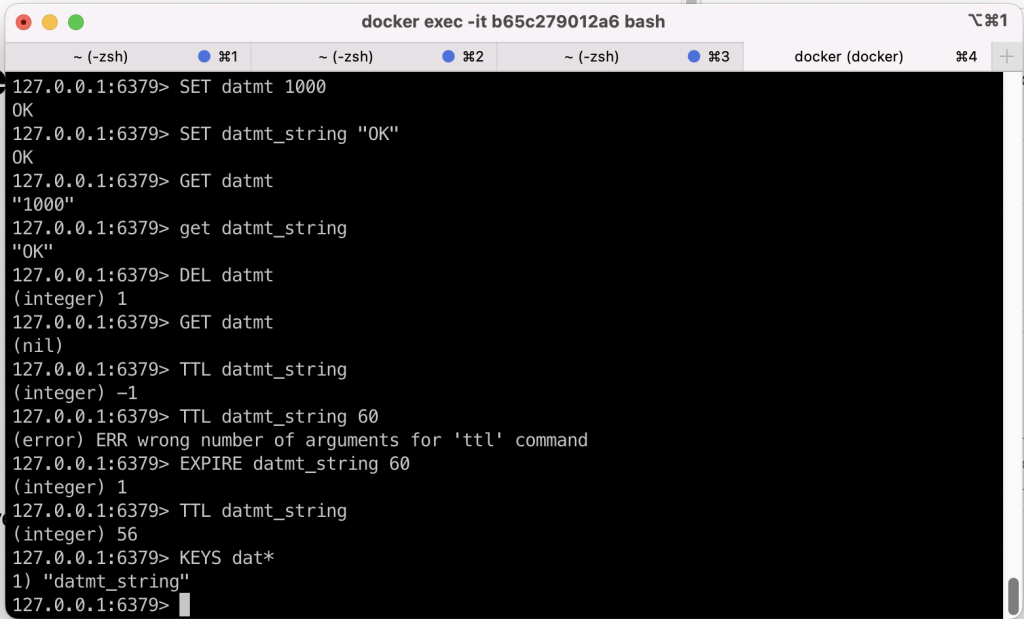
String Commands
APPEND key value #: append a value to a key STRLEN key #: get the length of the value of a key INCR key #: increment the value of a key by 1 DECR key #: decrement the value of a key by 1 INCRBY key increment #: increment the value of a key by a specified amount DECRBY key decrement #: decrement the value of a key by a specified amount
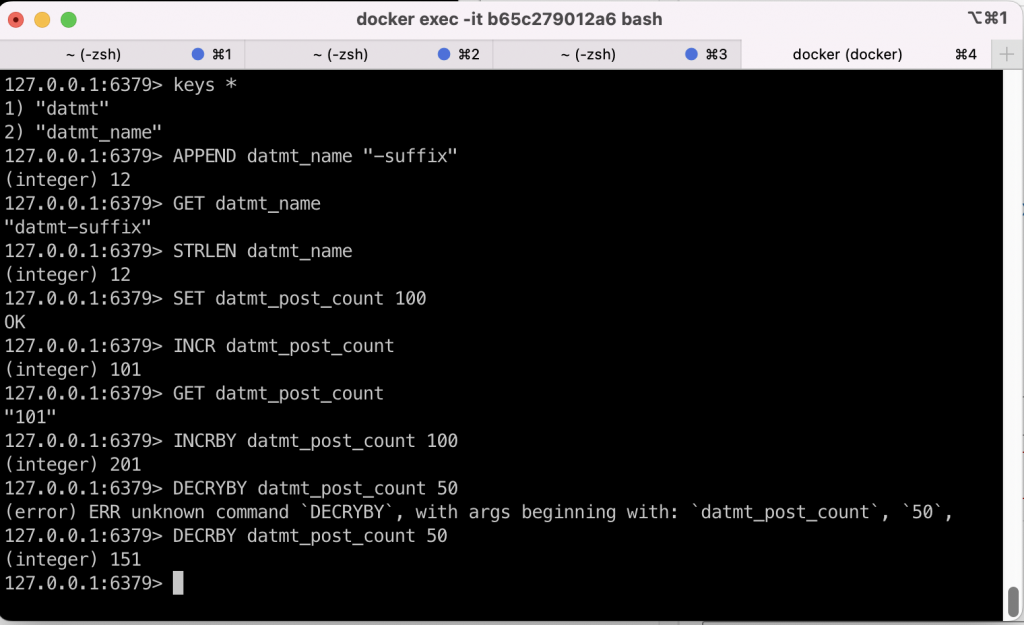
Hash Commands
HSET key field value #: set a field in a hash to a value HGET key field #: get the value of a field in a hash HDEL key field [field …] #: delete one or more fields from a hash HGETALL key #: get all fields and values from a hash HKEYS key #: get all fields from a hash HVALS key #: get all values from a hash HEXISTS key field #: check if a field exists in a hash HLEN key #: get the number of fields in a hash
Here is an example of the operation on an actual hash:
# Initialize the hash HMSET user name "John Doe" age 30 email "[email protected]" # Get the value of a field HGET user name # Output: "John Doe" # Get the values of multiple fields HMGET user name age # Output: 1) "John Doe" 2) "30" # Get all fields and values HGETALL user # Output: 1) "name" 2) "John Doe" 3) "age" 4) "30" 5) "email" 6) "[email protected]" # Get all fields HKEYS user # Output: 1) "name" 2) "age" 3) "email" # Get all values HVALS user # Output: 1) "John Doe" 2) "30" 3) "[email protected]" # Get the number of fields HLEN user # Output: 3 # Modify a field HSET user age 31 HGET user age # Output: "31" # Increment a field HINCRBY user age 1 HGET user age # Output: "32" # Delete a field HDEL user email HKEYS user # Output: 1) "name" 2) "age"
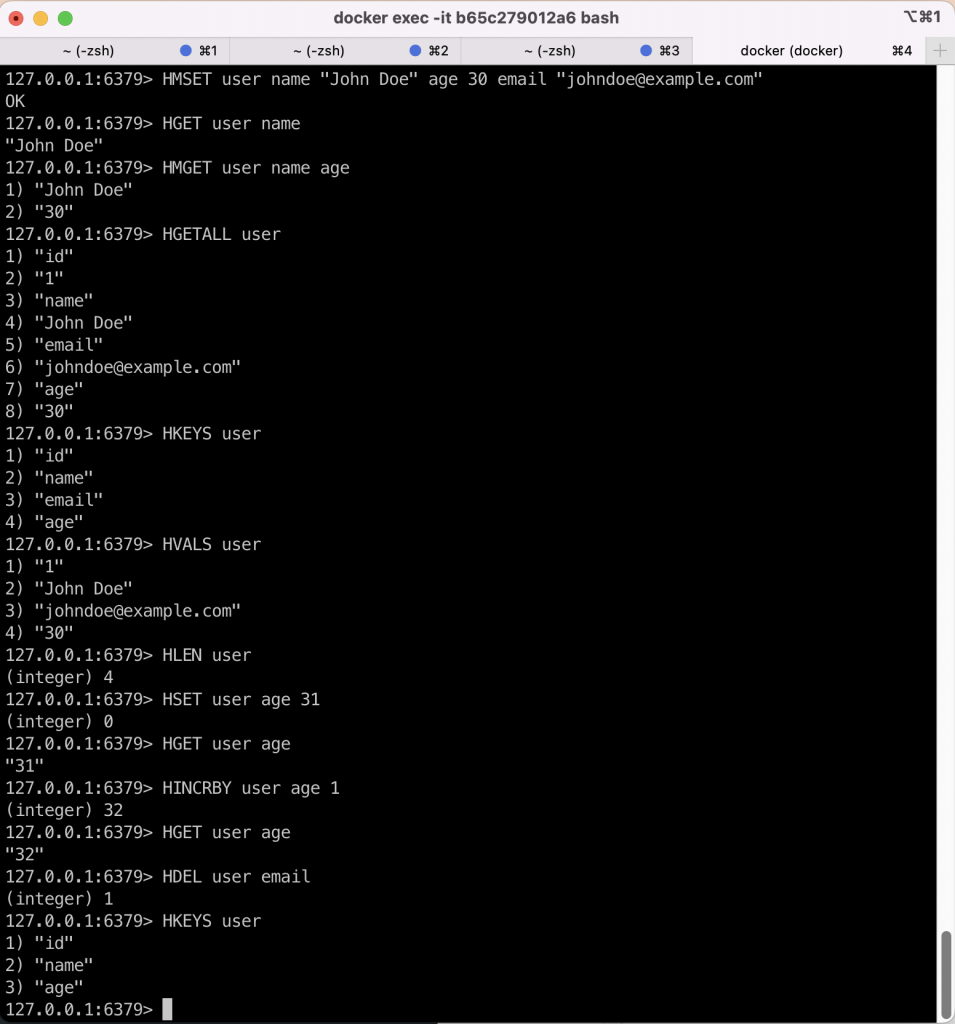
List Commands
LPUSH key value [value …] #: prepend one or more values to a list RPUSH key value [value …] #: append one or more values to a list LPOP key #: remove and return the first element of a list RPOP key #: remove and return the last element of a list LINDEX key index #: get the element at a specified index in a list LLEN key #: get the length of a list LRANGE key start stop #: get a range of elements from a list LREM key count value #: remove a specified number of occurrences of a value from a list
Let’s try the commands on an actual list:
# Initialize the list LPUSH numbers 3 2 1 # Get the length of the list LLEN numbers # Output: 3 # Get an element by index LINDEX numbers 1 # Output: "2" # Get a range of elements LRANGE numbers 0 1 # Output: 1) "1" 2) "2" # Append an element RPUSH numbers 4 LRANGE numbers 0 -1 # Output: 1) "1" 2) "2" 3) "3" 4) "4" # Pop an element LPOP numbers # Output: "1" RPOP numbers # Output: "4" LRANGE numbers 0 -1 # Output: 1) "2" 2) "3" # Insert an element LINSERT numbers BEFORE 2 1 LRANGE numbers 0 -1 # Output: 1) "1" 2) "2" 3) "3" # Remove an element LREM numbers 1 2 LRANGE numbers 0 -1 # Output: 1) "1" 2) "3" # Trim the list LTRIM numbers 0 0 LRANGE numbers 0 -1 # Output: 1) "1"
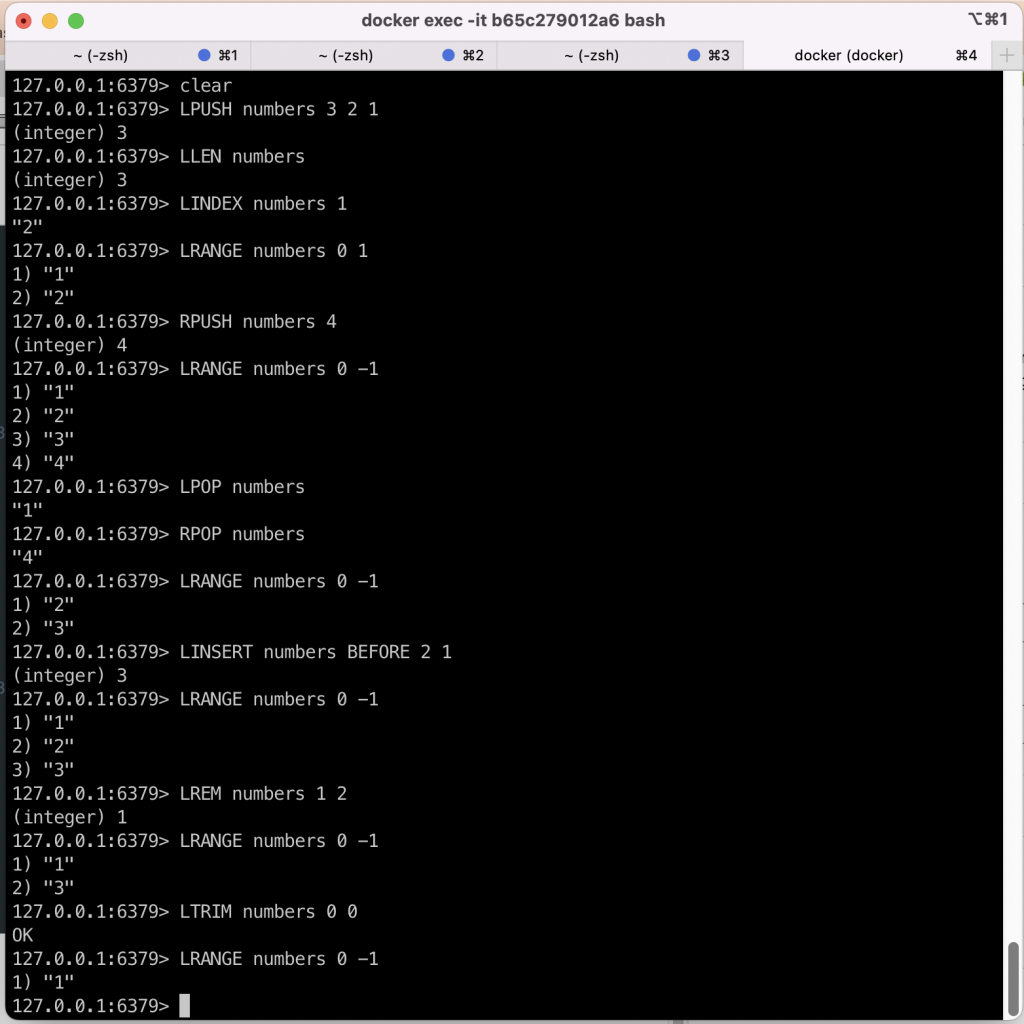
Set Commands
SADD key member [member …] #: add one or more members to a set SREM key member [member …] #: remove one or more members from a set SMEMBERS key #: get all members of a set SISMEMBER key member #: check if a member exists in a set SINTER key [key …] #: get the intersection of sets SUNION key [key …] #: get the union of sets
Here are the commands on an actual set. Notice that since set is not an ordered datatype, the order of items when you run the following commands could be different:
# Initialize the set SADD fruits apple banana orange # Get the number of elements in the set SCARD fruits # Output: 3 # Check if an element is a member of the set SISMEMBER fruits apple # Output: 1 (true) # Get all members of the set SMEMBERS fruits # Output: 1) "apple" 2) "banana" 3) "orange" # Remove an element from the set SREM fruits banana SMEMBERS fruits # Output: 1) "apple" 2) "orange" # Add multiple elements to the set SADD fruits pear peach SMEMBERS fruits # Output: 1) "apple" 2) "orange" 3) "pear" 4) "peach" # Get the intersection of multiple sets SADD fruits2 orange kiwi SINTER fruits fruits2 # Output: 1) "orange" # Get the union of multiple sets SUNION fruits fruits2 # Output: 1) "apple" 2) "orange" 3) "pear" 4) "peach" 5) "kiwi" # Get a random element from the set SRANDMEMBER fruits # Output: "pear"
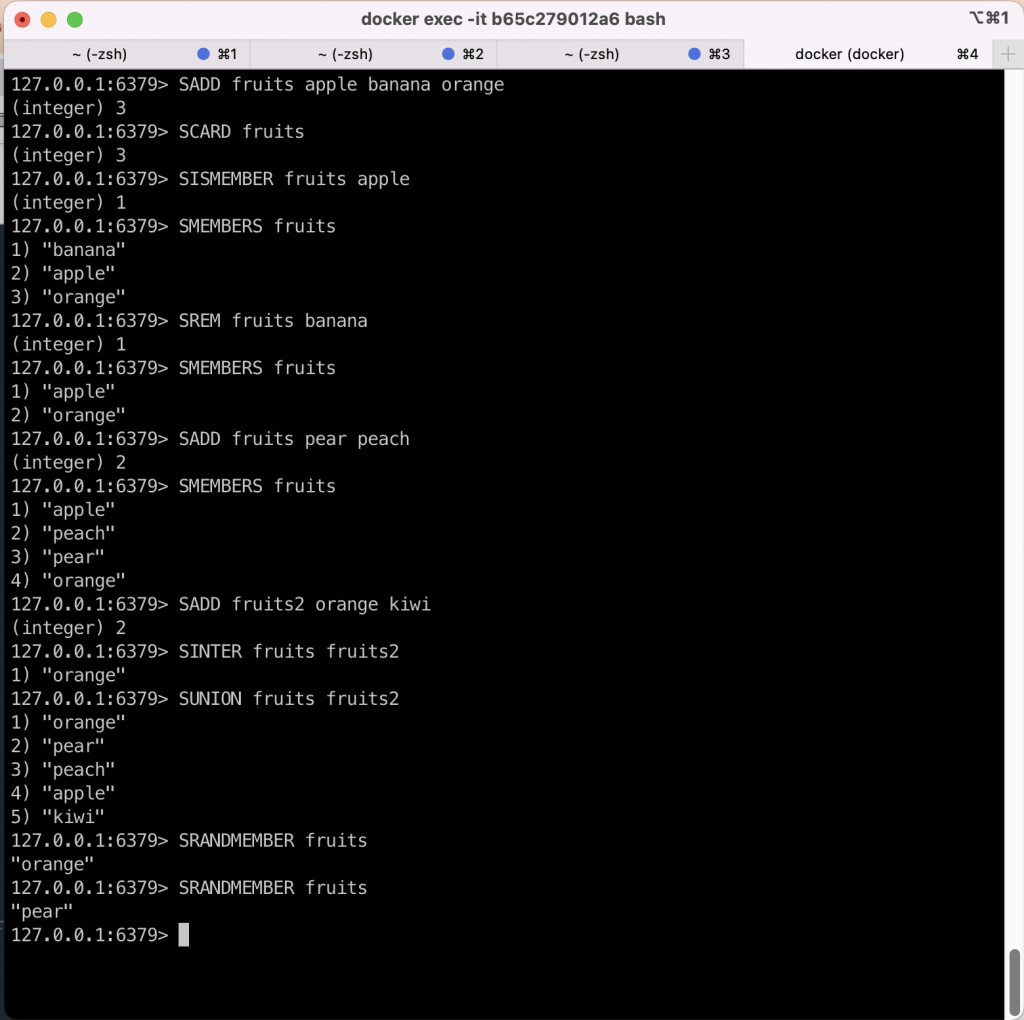
Sorted Set Commands
ZADD key score member [score member …] #: add one or more members with scores to a sorted set ZREM key member [member …] #: remove one or more members from a sorted set ZRANGE key start stop [WITHSCORES] #: The order of elements is from the lowest to the highest score. Elements with the same score are ordered lexicographically. ZREVRANGE key start stop [WITHSCORES] #: Returns the specified range of elements in the sorted set stored at key. The elements are considered to be ordered from the highest to the lowest score. ZSCORE key member #: get the score of a member in a sorted set
Here are the commands on an actual sorted set:
# Initialize the sorted set ZADD scores 90 "Alice" 85 "Bob" 95 "Charlie" # Get the number of elements in the sorted set ZCARD scores # Output: 3 # Get the rank of an element ZRANK scores "Charlie" # Output: 2 # Get the score of an element ZSCORE scores "Bob" # Output: 85 # Get a range of elements by rank ZRANGE scores 0 1 # Output: 1) "Bob" 2) "Alice" # Get a range of elements by score ZRANGEBYSCORE scores 90 95 # Output: 1) "Alice" 2) "Charlie" # Increment the score of an element ZINCRBY scores 10 "Alice" ZSCORE scores "Alice" # Output: 100 # Remove an element from the sorted set ZREM scores "Bob" ZRANGE scores 0 -1 # Output: 1) "Charlie" 2) "Alice" # Get the highest-scoring element(s) ZREVRANGE scores 0 0 # Output: 1) "Alice"
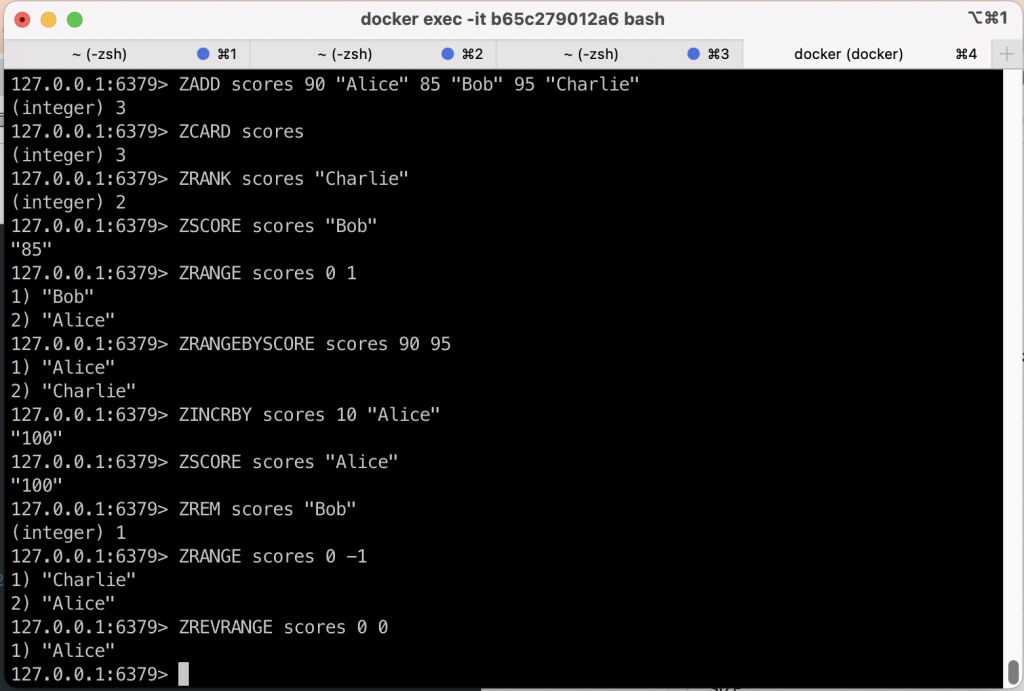
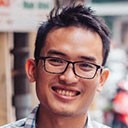
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.