Welcome to Day 4 of our Python learning journey! In this lesson, we will explore two important data types in Python: dictionaries and tuples.
Dictionaries
A dictionary is a collection of key-value pairs, where each key maps to a specific value. Dictionaries are created using curly braces {}
, with keys and values separated by colons :
. Here’s an example:
# Creating a dictionary of student grades grades = { "Alice": 85, "Bob": 92, "Charlie": 78, "Dave": 90 }
In this example, the keys are the names of the students, and the values are their corresponding grades. You can access a specific value in a dictionary by using its key in square brackets []
. For example:
# Accessing a value in a dictionary print("Bob's grade is: " + str(grades["Bob"]))
Dictionaries also have several built-in methods that allow you to manipulate or retrieve data from them. Some of the most commonly used methods include:
keys()
: returns a list of all the keys in the dictionaryvalues()
: returns a list of all the values in the dictionaryitems()
: returns a list of all the key-value pairs in the dictionary as tuples
print("Keys:") print(grades.keys()) print("Values:") print(grades.values()) print("Dictionary Items:") for item in grades.items(): print(item)
Here is the output:
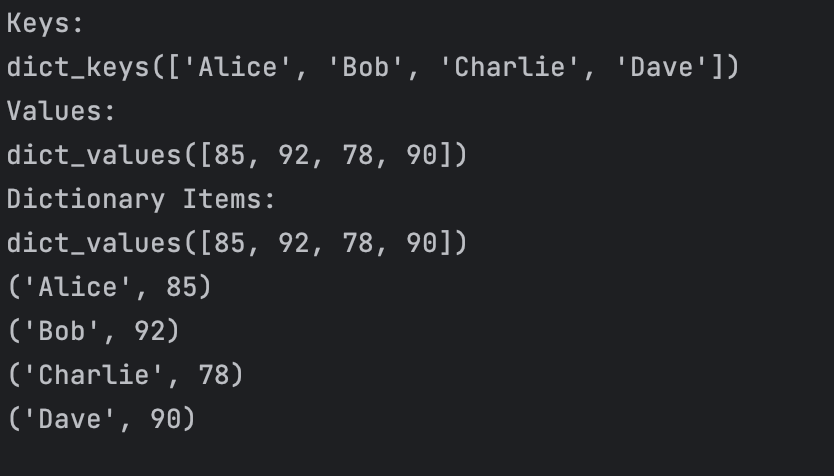
As you can see, using keys
and values
operations on a dictionary produce lists. The items
operation produces a list of Tuples, which we will discover in the next section.
Tuples
A tuple is an ordered, immutable collection of values. Tuples are created using parentheses ()
, with values separated by commas. Here’s an example:
# Creating a tuple of coordinates coordinates = (3.14, 2.71)
The tuple here contains two values representing the x and y coordinates. You can access a specific value in a tuple using indexing, starting from 0. For example:
# Accessing a value in a tuple print(coordinates[0]) # 3.14
Because tuples are immutable, you cannot modify their values once they are created. However, you can use several built-in methods to perform various operations on tuples. Some of the most commonly used methods include:
count()
: returns the number of times a specific value appears in the tupleindex()
: returns the index of the first occurrence of a specific value in the tuple
my_favorite_food = ("Banana", "Apple", "Bread") print(my_favorite_food.count("Pine")) #0 because Pine is not in the tuple print(my_favorite_food.index("Apple")) #1
As you can see, you can use count to check if an item is in the tuple. It’s quite tempting to use the index to check for an item’s existence. However, if the item is not in the tuple, an error will throw.
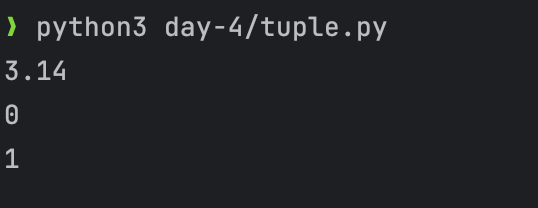
List, Tuple & Dictionary comparison
Now you’ve learned List, Tuple and Dictionary. Let’s compare these data structures:
Characteristic | List | Tuple | Dictionary |
---|---|---|---|
Mutable/Immutable | Mutable | Immutable | Mutable |
Order | Ordered | Ordered | Unordered |
Indexing | Uses integer index | Uses integer index | Uses key |
Duplicate Values | Allowed | Allowed | Not allowed |
Syntax | [ ] | ( ) | { } |
Modification | Can be modified | Cannot be modified | Can be modified |
Iteration | Slower than tuple | Faster than list | Slower than list |
Size | Takes more memory | Takes less memory | Takes more memory |
Use cases | When you need to modify data | When you need to access data | When you need to access by keys |
In summary, lists are mutable, ordered, and allow duplicate values, tuples are immutable, ordered, and allow duplicate values, and dictionaries are mutable, unordered, and do not allow duplicate keys.
Tuple iteration is typically faster than dictionary and list iteration in Python because tuples are immutable, which means they cannot be modified after they are created. This allows Python to optimize the iteration process by using a more efficient memory layout that can be processed faster by the CPU.
When you iterate over a tuple, Python can store the entire tuple in a contiguous block of memory, which can be accessed very quickly by the CPU. In contrast, when you iterate over a list or dictionary, the elements may be scattered throughout memory, which can slow down the iteration process.
Additionally, dictionaries use a hash table to store their key-value pairs, which can introduce some overhead when iterating over them. Lists also need to dynamically resize as elements are added or removed, which can cause memory fragmentation and slow down iteration.
Overall, while there are certainly cases where lists or dictionaries may be more appropriate for a particular use case, tuples can be a good choice when you need to iterate over a collection of elements as quickly as possible.
In terms of memory usage, tuples, and lists are similar since they are both implemented as dynamic arrays. However, tuples are generally more memory efficient because they are immutable, meaning that their size and content cannot be changed after they are created. This allows for more efficient memory allocation.
Dictionaries, on the other hand, have a larger memory footprint because they are implemented using a hash table. This means that they require more overhead to store the key-value pairs, as well as additional memory for collision resolution and hash table resizing. However, dictionaries offer fast lookups and insertions, especially for large datasets.
Conclusion
Now that you have a basic understanding of dictionaries and tuples in Python, it’s time to start practicing! Try creating your own dictionaries and tuples, and experiment with the built-in methods to see how they work. Good luck, and stay curious!
As usual, the code for this post is available here on GitHub
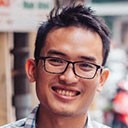
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.