Table of Contents
Introduction
Strings and Lists are the two most data structures in Python. In this post, we will learn all the essential things about these two topics.
String
In Python, a string is a sequence of characters enclosed in quotes. You can use either single quotes ('
) or double quotes ("
) to create a string. For example:
my_string = "Hello, world!"
Strings are immutable in Python, meaning you cannot modify a string once it has been created. However, you can perform operations on strings that create new strings.
Common built-in String methods
Here are the most common string-related methods you use as a Python developer
my_string = " Hello, world! " print(len(my_string)) # prints 15 print(my_string.lower()) # prints " hello, world! " print(my_string.upper()) # prints " HELLO, WORLD! " print(my_string.strip()) # prints "Hello, world!" print(my_string.split()) # prints ["Hello,", "world!"] print("-".join(["Hi, ", "there"])) # prints "Hi, there"
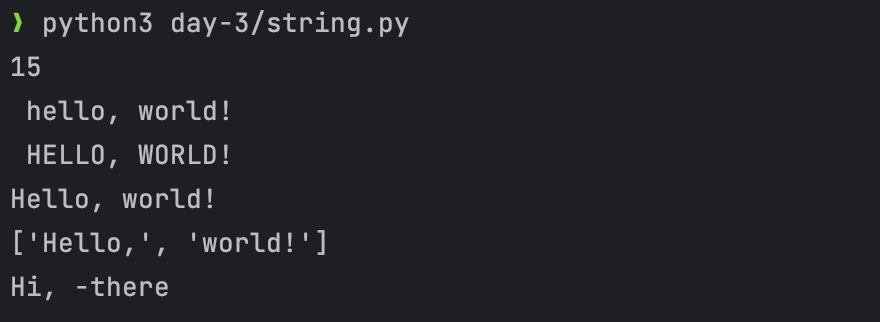
String concatenation & conversion
One of the most common operations in any programming language is string concatenation (which is the fancy word for joining strings).
In the example above, you have seen the method join
. However, you can join strings by using the plus (operator):
print("Hello, " + "there") # Hello, there
However, when you have other data types, you need to convert them to strings using the str method first before concatenating them with other strings:
# Converting an integer to a string num = 123 num_str = str(num) print(num_str) # "123" # Converting a float to a string pi = 3.14159 pi_str = str(pi) print(pi_str) # "3.14159" # Converting a boolean to a string flag = True flag_str = str(flag) print(flag_str) # "True" # Converting a list to a string my_list = [1, 2, 3] list_str = str(my_list) print(list_str) # "[1, 2, 3]"
Note that when you convert other data types to strings, the resulting string will always be enclosed in quotes. In addition, you may want to keep in mind that some data types may not be easily convertible to strings, or may require additional formatting or manipulation to get the desired string representation.
Lists
In Python, a list is a collection of items enclosed in square brackets ([]
). Each item in the list can be of any data type, including other lists. For example:
my_list = [1, 2, 3, "four", ["five", "six"]] # you can print the list using print print(my_list)

Common list methods
Here are some important built-in methods you can do with lists in Python:
my_list = [1, 2, 3, 4, 5] print(len(my_list)) # returns the number of items in a list => prints 5 my_list.append(6) # adds an item to the end of a list print(my_list) # prints [1, 2, 3, 4, 5, 6] my_list.insert(2, "three") # inserts an item at a specified position in a list. print(my_list) # prints [1, 2, "three", 3, 4, 5, 6] my_list.remove(3) # removes the first occurrence of an item from a list. print(my_list) # prints [1, 2, "three", 4, 5, 6] item = my_list.pop(3) # removes and returns the item at a specified position in a list. print(my_list) # prints [1, 2, "three", 5, 6] print(item) # prints 4 integer_list = [4, 3, 2, 0] integer_list.sort() print(integer_list) # print [0, 2, 3, 4] my_list.sort() # throws error print(my_list) #
Additional lists operations
Some additional operations would benefit greatly if you know:
Nested list
# Creating a nested list my_list = [[1, 2], [3, 4], [5, 6]] # Accessing an item in a nested list print(my_list[1][0]) # Output: 3
Looping through a list
# Looping through a list my_list = [1, 2, 3] for item in my_list: print(item)
List comprehension
# Using list comprehension to create a new list my_list = [1, 2, 3] new_list = [item * 10 for item in my_list] print(new_list) # Output: [10, 20, 30]
Conclusion
In this post, we’ve learned some of the most important string and list operations. In the next lesson, you will learn about two more useful data structures: Tuple and Dictionary
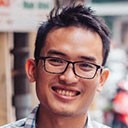
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.