Table of Contents
Overview
In this post, we are going to learn how to create a Jenkins pipeline to deploy the Angular app to our server.
If you prefer to watch the video tutorial instead, here it is:
Creating the configuration files
To deploy the Angular app, we need to have a Dockerfile and an nginx.conf file since the static files will be deployed using nginx.
The nginx.conf file
server { listen 80; server_name localhost; gzip on; gzip_types text/plain application/xml; gzip_proxied no-cache no-store private expired auth; gzip_min_length 1000; root /usr/share/nginx/html; index index.html; location / { try_files $uri $uri/ /index.html; } error_page 500 502 503 504 /50x.html; location = /50x.html { root /usr/share/nginx/html; } }
The Dockerfile
FROM nginx:1.23.1-alpine ARG PIGGYS_BUILD_SHA COPY nginx.conf /etc/nginx/conf.d/default.conf COPY /dist/wallet-ui /usr/share/nginx/html RUN grep -rl "PIGGYS_BUILD_SHA" /usr/share/nginx/html | xargs sed -i "s/PIGGYS_BUILD_SHA/${UK_BUILD_SHA}/g" && grep -rl "PIGGYS_BUILD_TIMESTAMP" /usr/share/nginx/html | xargs sed -i "s/PIGGYS_BUILD_TIMESTAMP/$(date '+%Y-%B-%d %H:%M:%S %Z')/g"
The Jenkinsfile
//this build push the latest from master to the PROD env //Build to prod ONLY trigger manually pipeline { agent any environment { DEPLOY_COMMAND="ssh -o StrictHostKeyChecking=no -p 25782 [email protected] echo PIGGYS_FE_VERSION=${GIT_COMMIT} > /data/other-projects/piggys-wallet/.env && docker-compose -f /data/other-projects/piggys-wallet/piggys-frontend.yaml up -d" DOCKERFILE="Dockerfile" SHORT_COMMIT = "${GIT_COMMIT[0..7]}" SSH_AGENT="jenkins_agent_prod" } stages { stage('Build image and push to docker hub') { steps { script { nodejs(nodeJSInstallationName: 'nodejs18') { sh 'corepack enable' sh 'corepack prepare pnpm@latest-8 --activate' sh 'pnpm install' sh 'pnpm build:prod' } echo "Build number ${GIT_COMMIT}" docker.withRegistry('https://registry.openexl.com/repository/d1', 'jenkins_docker') { def app = docker.build("piggys-wallet-frontend:${GIT_COMMIT}", "--build-arg PIGGYS_BUILD_SHA=${SHORT_COMMIT} -f ${DOCKERFILE} .").push() } } } } stage('Update deployment') { steps { script { echo "Deploy command: ${GIT_COMMIT} ${DEPLOY_COMMAND}" sshagent (credentials: ["${SSH_AGENT}"]) { sh '${DEPLOY_COMMAND}' } } } } } }
There is quite a lot happening in the Jenkinsfile here.
On line 7, I specified a deploy command. This is simply an SSH command to write the commit SHA to an .env file and then run docker compose up to deploy the application.
There are two stages, the first is to build a docker image and push it to the remote repository. The second stage is to run the deploy command, which will pull the newly built docker image and deploy it.
The piggys-frontend.yaml file
This is the file on the server where the docker-compose command would pick and run as in the DEPLOY_COMMAND script:
version: '3' services: piggys-wallet-frontend: container_name: piggys-wallet-frontend image: registry.openexl.com/piggys-wallet-frontend:${PIGGYS_FE_VERSION} restart: always networks: - sso-net networks: sso-net: external: true
Configure a Jenkins Pipeline to deploy
Now let’s configure a Jenkins pipeline to deploy the Angular application to the server:
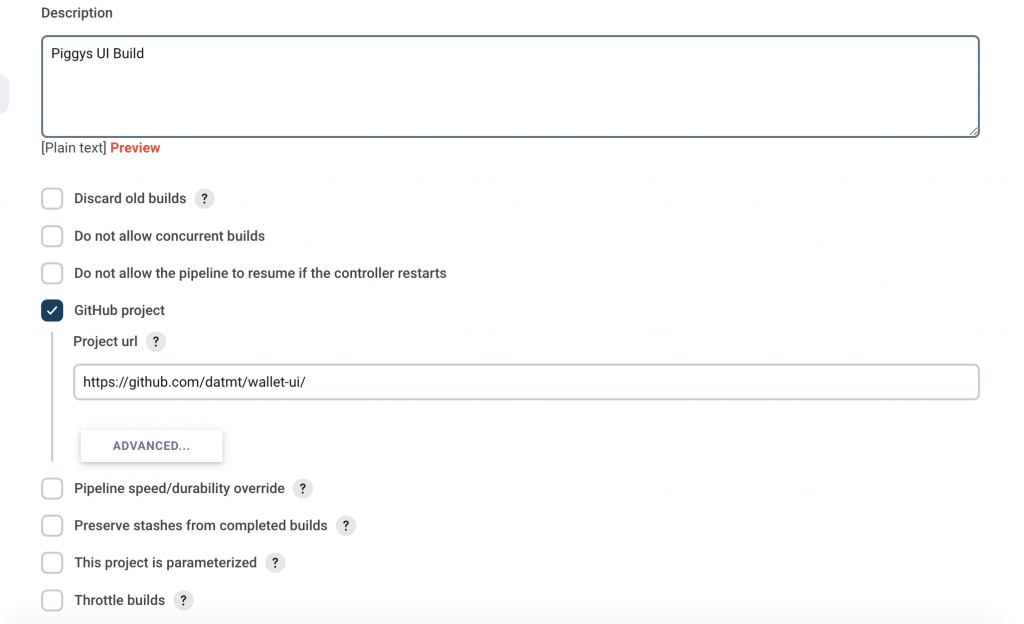
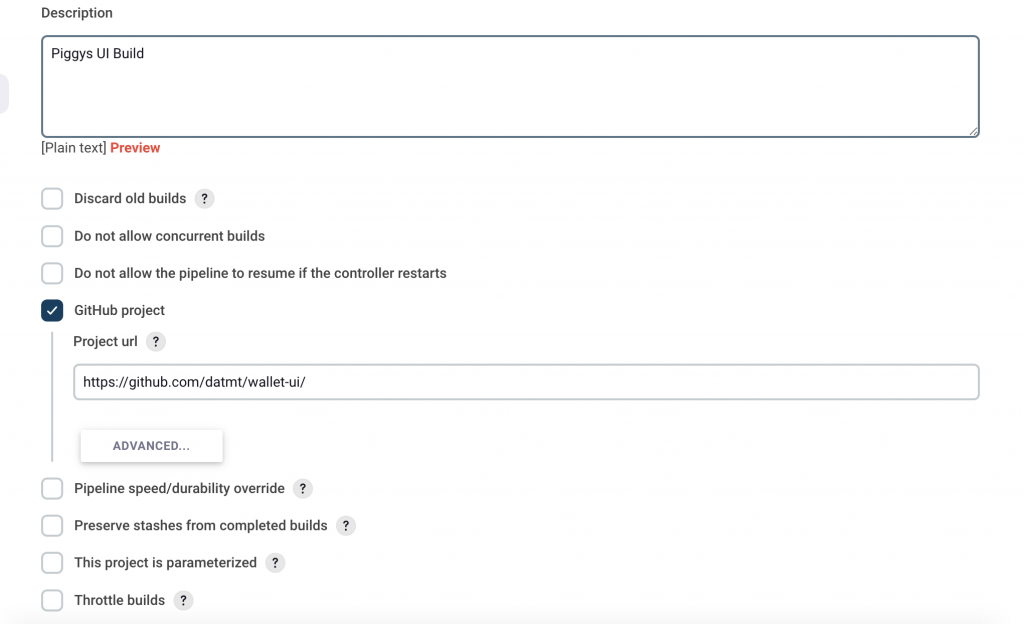
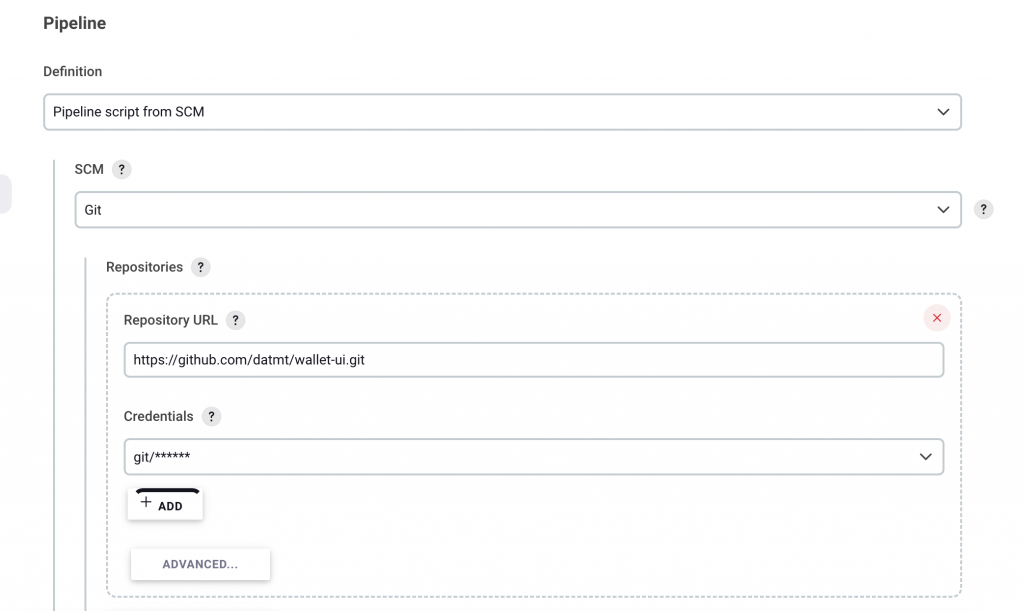
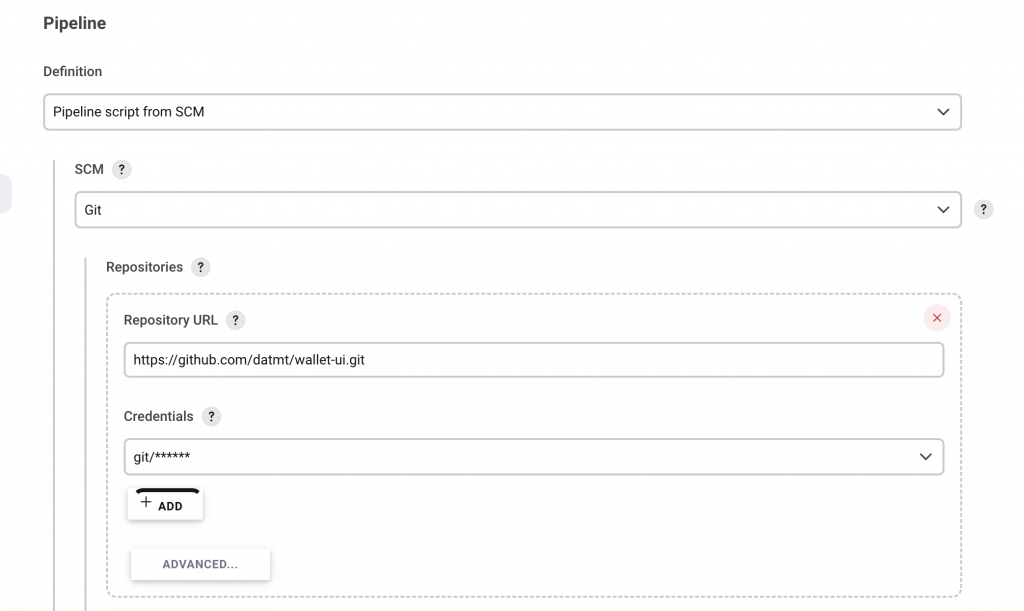
Here, I configured the pipeline to use the GitHub project. However, this is not sufficient to guarantee an auto build yet.
Now, let’s go to the GitHub Repository to configure the Jenkins webhook so every time we push to GitHub, there is an event sent to Jenkins to trigger the build.
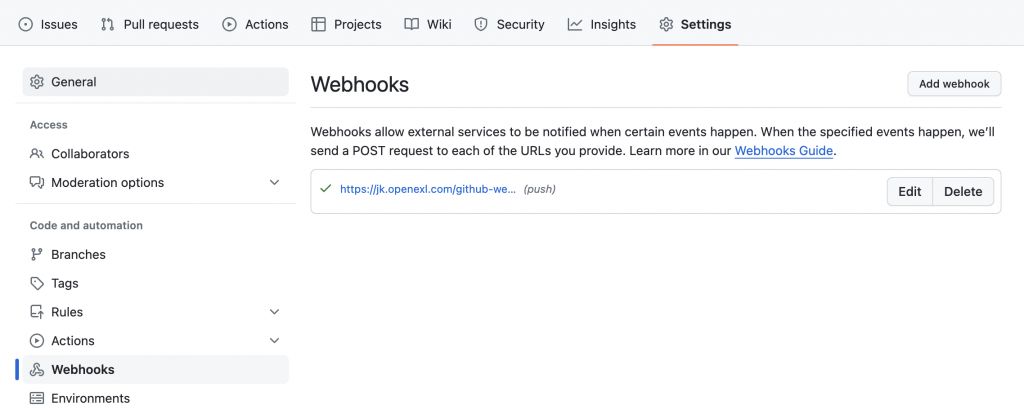
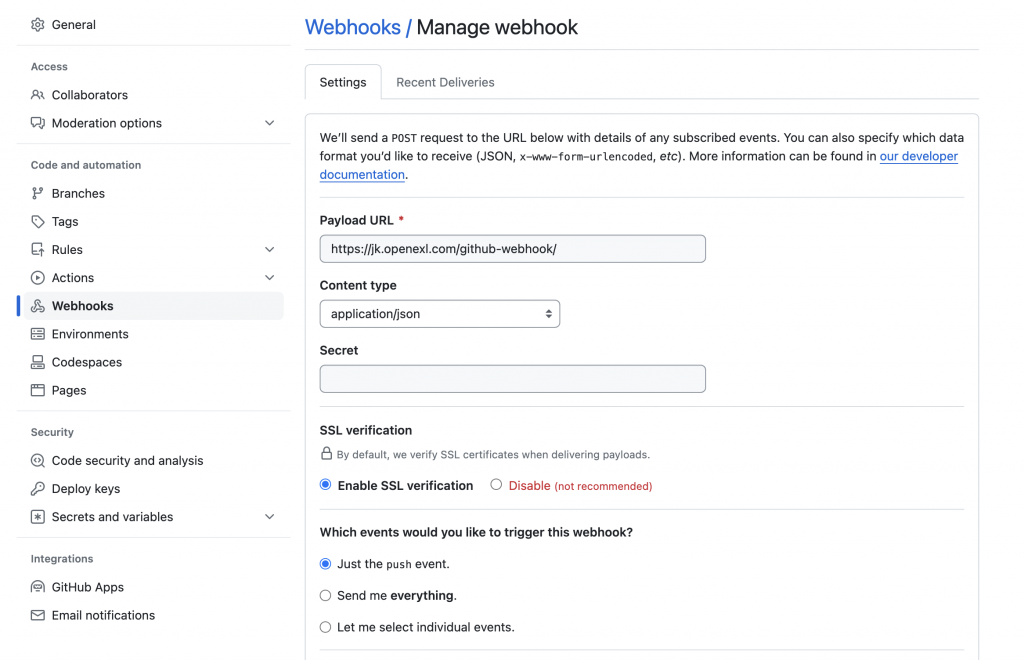
The WebHook URL is the Jenkins host URL + /github-webhook/
Make sure to save the changes and back to Jenkins.
There is one big caveat with Jenkins auto build trigger: You need to trigger a build manually first. Only after that, new pushes to the Git repo will trigger an auto build.
I learned this the hard way.
Viewing the Pipe line Running
If you configure the pipeline correctly and make a manual run first, you should be able to see auto build in action in subsequent pushes:
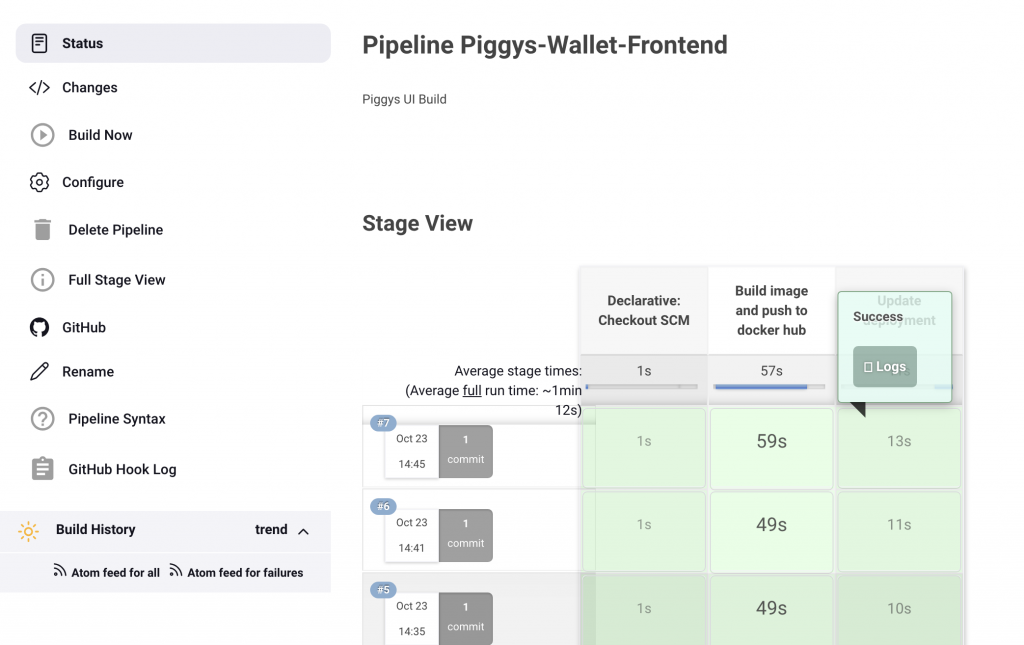
Conclusion
In this post, we have configured the Jenkins pipeline to build and deploy our Angular app to the server. In the next post, we are going to do the same with the API
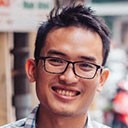
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.