Table of Contents
Labels are key, value pairs that help identify a particular object (pods/deployment…)
Working with labels and pod
Create a pod with labels
# Create pod kubectl run my-pod1 --image=nginx --labels="type=webserver,image-name=nginx" # Get all labels of a pod kubectl get pod my-pod1 --show-labels # Output NAME READY STATUS RESTARTS AGE LABELS my-pod1 1/1 Running 0 44s image-name=nginx,type=webserver
Add labels to running pods
# add a label kubectl label pod my-pod1 opensource=true # add multiple labels kubectl label pod my-pod1 a=1 b=2 # Get the labels kubectl get pod my-pod1 --show-labels # Output NAME READY STATUS RESTARTS AGE LABELS my-pod1 1/1 Running 0 3m25s a=1,b=2,image-name=nginx,opensource=true,type=webserve
Update an existing label
# Update current label (previously, a=1) kubectl label pod my-pod1 a=2 --overwrite=true # Get pod labels kubectl get pod my-pod1 --show-labels # Output NAME READY STATUS RESTARTS AGE LABELS my-pod1 1/1 Running 0 4m56s a=2,b=2,image-name=nginx,opensource=true,type=webserver
If you prefer, setting labels in YAML file is possible under metadata section.
Select a pod based on its label
Let’s create a few more pods with various labels
kubectl run my-pod2 --image=nginx --labels="type=webserver,duty=backup,ver=1.0.0"
Now, we can use --selector
to select pods:
kubectl get pods --selector="type=webserver" # Output NAME READY STATUS RESTARTS AGE my-pod1 1/1 Running 0 9m49s my-pod2 1/1 Running 0 33s kubectl get pods --selector="duty=backup" # Output, only my-pod2 is shown since only this pod has that label NAME READY STATUS RESTARTS AGE my-pod2 1/1 Running 0 87s
We can omit the value (after the =
sign) to get the objects that have that label, regardless of the values
kubectl get pods --selector="a" # Output NAME READY STATUS RESTARTS AGE my-pod1 1/1 Running 0 12m kubectl get pods --selector="type" #Output NAME READY STATUS RESTARTS AGE my-pod1 1/1 Running 0 13m my-pod2 1/1 Running 0 4m17s pod-with-label 1/1 Running 0 106m
Finally, we can select objects that have value exists in a possible set. First of all, get all pods and show their labels
# First get all pods and show their labels: kubectl get pods --show-labels # Output NAME READY STATUS RESTARTS AGE LABELS my-pod1 1/1 Running 0 15m a=2,b=2,image-name=nginx,opensource=true,type=webserver my-pod2 1/1 Running 0 6m43s duty=backup,type=webserver,ver=1.0.0 my-pod3 1/1 Running 0 9s duty=backup,type=proxy,ver=1.0.0 nginx 1/1 Running 0 33h run=nginx pod-with-label 1/1 Running 0 108m image=new-nginx,type=server pod2 0/1 CreateContainerConfigError 0 5d15h <none> pod2-player 1/1 Running 3 5d9h <none> pod3-player 1/1 Running 3 5d8h <none> pvpod1 1/1 Running 1 2d9h <none>
As you can see, my-pod1
, my-pod2
, my-pod3
all have label type
. If we want to select the ones with type webserver
or server
, we can run the following
kubectl get pods --selector="type in (webserver,server)" # Output NAME READY STATUS RESTARTS AGE my-pod1 1/1 Running 0 18m my-pod2 1/1 Running 0 9m11s pod-with-label 1/1 Running 0 111m
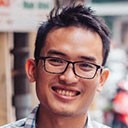
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.