Table of Contents
Overview
Recently, I needed to add a feature to my gotkey.io application that lets user stores key-value pairs for their product. The sensible data structure to use in this case is obviously, a map. While that worked perfectly on the backend side, the frontend side posed some challenges. Specifically, when I tried to send a Map over HTTP, none of the pairs were sent.
Let’s see how I managed to overcome this issue.
The fix
It turned out, that using a normal object ({}) worked fine. Let’s see the code:
export interface Product { //... properties?: {[key: string]: string}; //... }
Adding and removing key value pairs to the property is quite simple too:
addProperty(newPropertyKey: string, newPropertyValue: string) { //initialize properties if not initialized if (!this.product.properties) { this.product.properties = {}; } if (Object.keys(this.product.properties).indexOf(newPropertyKey) !== -1) { return; } this.product.properties[`${newPropertyKey}`] = newPropertyValue; }
Remove property:
deleteProperty(key: string) { delete this.product.properties![key]; }
With this data structure, you can send the property over HTTP requests without issues.
Conclusion
In this post, I’ve provided an example of overcoming the limitation of sending TypeScript Map objects over HTTP by using javascript object literal.
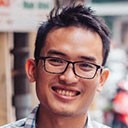
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.