Table of Contents
What is a thread?
Thread is the smallest execution unit that can be schedule by the operating system.
A process is a running program. When you start a program, you create a process.
In one process, there can be more than one thread.
Threads in a process share an environment created by the process.
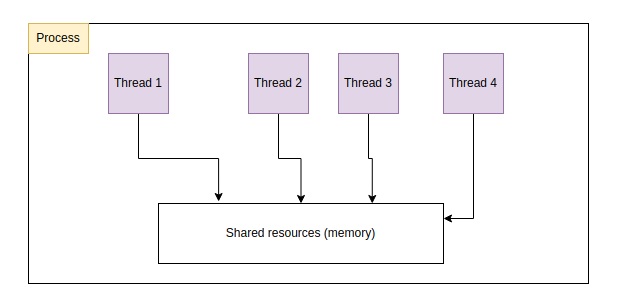
System vs user-defined threads
All java applications are multi-threaded. There are threads created by the developer and threads created by the system.
Garbage collection is one example of thread created by the system. In the other hand, if your application doing something, for example processing image, there is a thread for that task. It is the one created by you, the developer.
Thread scheduler
Most of the time, there are more tasks that the number of CPU core. Thus, it is neccessary for the CPU to schedule
when to run which task. This scheduling is handled by the thread scheduler.
Context switching
Context switching happens when one CPU core has to stop running one task and switch to running other task. One possible
reason is, for example, task A is allocated a time segment of 10ms. After 10ms, even if task A hasn’t finished yet, the
thread scheduler need to switch to running other task.
Context switching is not free. It costs CPU resources to stop executing one task and start running other.
Thread priority
One thread can have priority from 1 to 10. The thread scheduler take this number into consideration to decide which
task to run.
In Java programming, by default, a thead has priority 5.
Consider this code:
public class D_Thread { public static void main(String[] args) { Thread t1 = new Thread(() -> System.out.println("Current thread priority: " + Thread.currentThread().getPriority())); t1.start(); } }
This is the result in console:
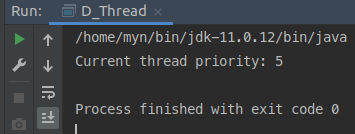
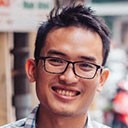
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.