Table of Contents
Declaring variables in Scala is quite simple. The language offers some very nice mechanisms to let developers declare variables with ease.
Mutable variables
To declare mutable variables (variables that you can assign to a different value after declaring), you need to use the keyword var
:
def mutableVariable(): Unit = var x = 100; println(s"x is: ${x}") x = 200 println(s"x is: ${x}"
Immutable variables
To declare immutable variables, instead of using var
, you need to use val
def immutableVariable(): Unit = val x = 100
Trying to assign x to another value would cause a compiler error:
def immutableVariable(): Unit = val x = 100 x = 100 //<- this would cause a compiler error
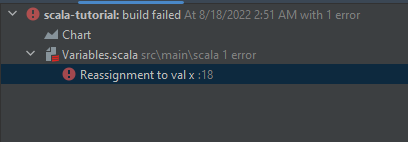
Type inference
As you can see from the examples above, I declared variables without specifying the types. This is not because Scala is a loosely typed language like Javascript. Instead, it infers the type based on the value you assign to the variables.
You can specify the type of the variables if you want to.
def typeInference(): Unit = val dog1 = GSD_Dog("Joe") println("type of dog1: " + dog1.getClass) val x : String = "hello" println("type of x: " + x.getClass) var m = 100f println("type of m: " + m.getClass) var n = 100 println("type of n: " + n.getClass)
Calling this function produces the following results:
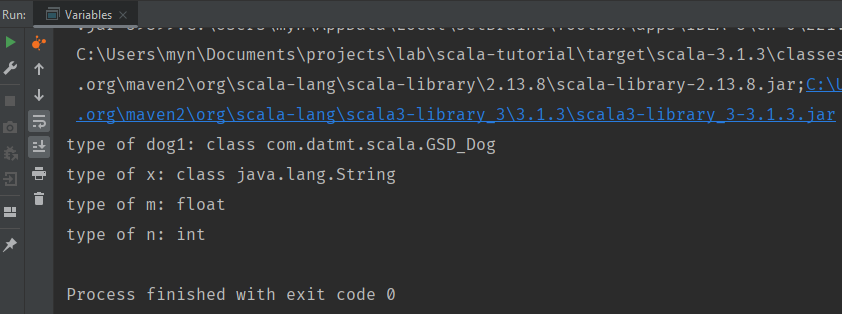
Multiple variables declaration
You can declare multiple variables (mutable and immutable on one line) like this:
def multipleVariableDeclaration(): Unit = var (x: Int, y: String, z: Boolean) = (100, "Hello", false) println(s"x = $x, y = $y, z = $z")
Calling the function would produce the correct values for x, y, z respectively:
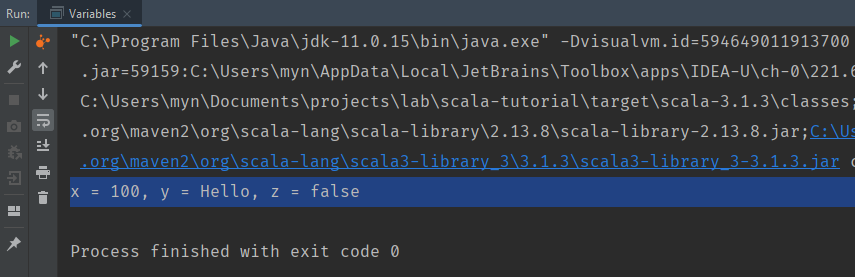
Variable scopes
There are three variable scopes in Scala:
- Field (class variable)
- Method parameters (arguments passed to methods)
- Local (variables declared inside methods)
Here is the example of the scopes:
case class GSD_Dog(name: String) { val breed: String = "GSD" def reportState(isSleeping: Boolean = true): Unit = var report = "Not sleeping" if (isSleeping) then report = "I'm busy" println(report) }
In this example,
-
breed
has field scope. isSleeping
has method parameters scopereport
has local variable scopes
Conclusion
In this post, I’ve introduced you to Scala variables. To recap, variables in Scala can be mutable or immutable. When declaring variables, you can specify their types or leave for type inference to do the work.
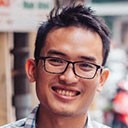
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.