Table of Contents
Overview
In the previous post, we integrated Angular with Auth0 successfully. In this post, we continue to integrate Auth0 with Spring Boot. The Spring Boot API is the resource server that our users will interact with to manage their wallets.
Also, as in the series introduction, we mentioned that the database we use would be MongoDB. This affects the choices of packages we include when configuring with Spring Initilizr.
Create a Spring Boot App with Spring Initializr
Let’s head over to https://start.spring.io to configure an application like this:
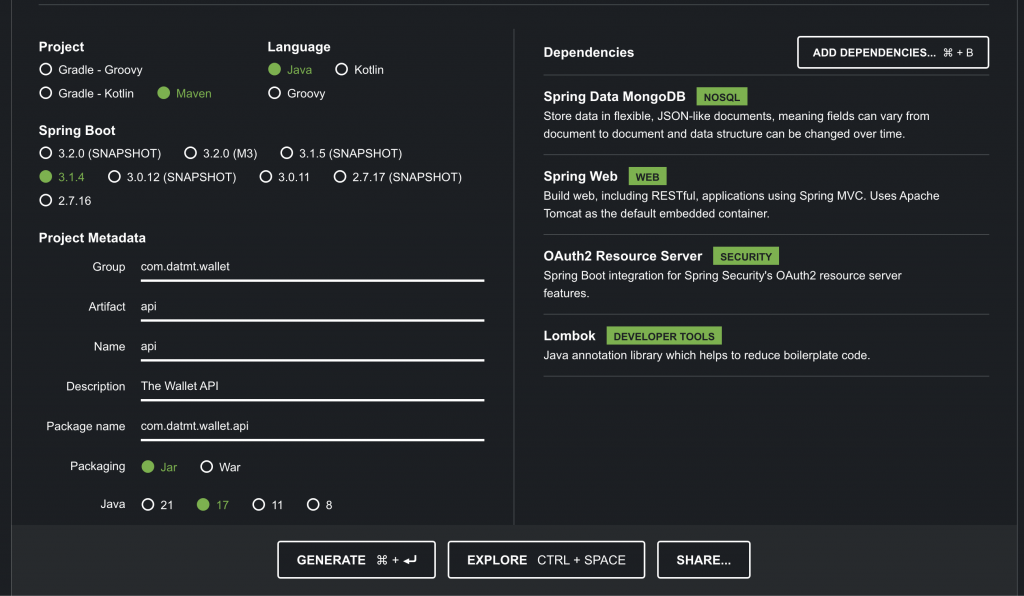
Since we haven’t setup MongoDB database yet, I’ll comment out the MongoDB dependency for now.
Now, we are ready to integrate Auth0 with Spring Boot.
Integrate Auth0 With Spring Boot
Let’s head over Auth0, login to your account, and select the application you created in the first post. Grab the domain:
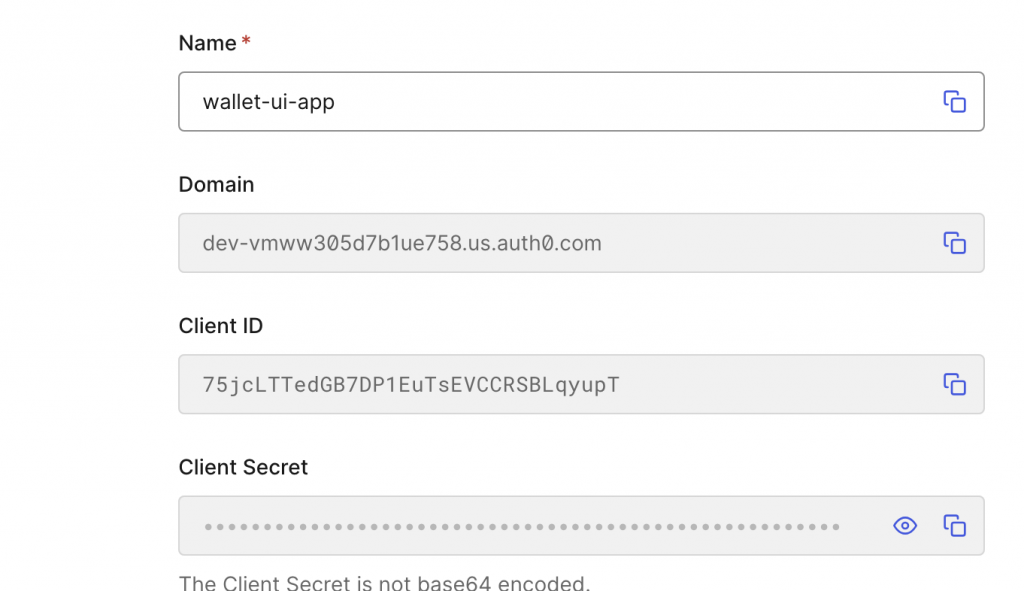
Next, fill your application.yml with the following details:
server: port: 9191 spring: security: oauth2: resourceserver: jwt: jwk-set-uri: https://dev-vmww305d7b1ue758.us.auth0.com/.well-known/jwks.json issuer-uri: https://dev-vmww305d7b1ue758.us.auth0.com/
Notice that on line 8, the URL is formed by using your URL and .well-known/jwks.json
Now let’s create a configuration class to project our endpoints:
@Configuration @EnableWebSecurity public class SecurityConfig { @Bean public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception { http .authorizeHttpRequests( authorizeHttpRequests -> authorizeHttpRequests .requestMatchers("/public/**").permitAll() .anyRequest().authenticated() ) .oauth2ResourceServer((oauth2) -> oauth2.jwt(Customizer.withDefaults())) .csrf(Customizer.withDefaults()); return http.build(); } }
This is all you need to secure the API endpoints. Notice that we allow the URLs that start with /public to be publicly accessible.
That’s all you need to do to integrate Auth0 with Spring Boot as a resource server.
Conclusion
In this post, we’ve integrated Spring Boot with Auth0. In the next post, we are going to test if the user can make a call from the Angular app to the backend API.
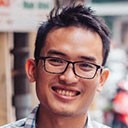
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.