Table of Contents
Overview
In the first two posts (integrating Auth0 with Angular and integrating Auth0 with Spring Boot) we have integrated Auth0 with both frontend and back end app. In this post, let’s try if we can use the token generated by Auth0 to call secured API endpoints from our Spring Boot app.
Let’s get started.
Create endpoints for the API backend
Let’s start by creating two endpoints, one is for public and one requires authentication.
Here is the public endpoint:
@RestController @RequestMapping("/public") public class PublicController { @GetMapping("/hello") public String hello() { return "Hello, world!"; } }
And here is the endpoint that requires authentication
@RestController @RequestMapping("/api/wallets") public class WalletController { @GetMapping() public String hello() { return "Hello, world!"; } }
Run the Spring Boot application, the public endpoint should be accessible without any authentication:
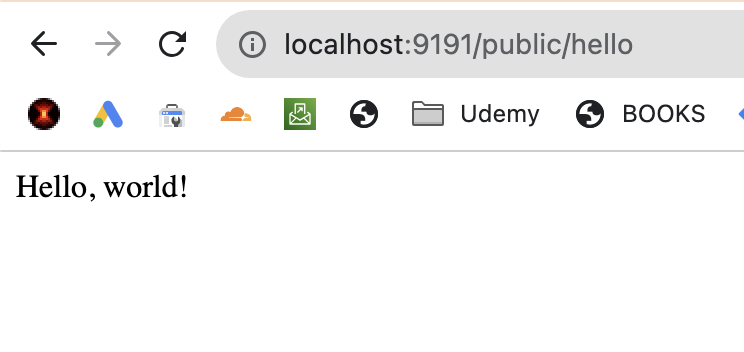
However, trying to access the wallet endpoint would result in a 401 error:
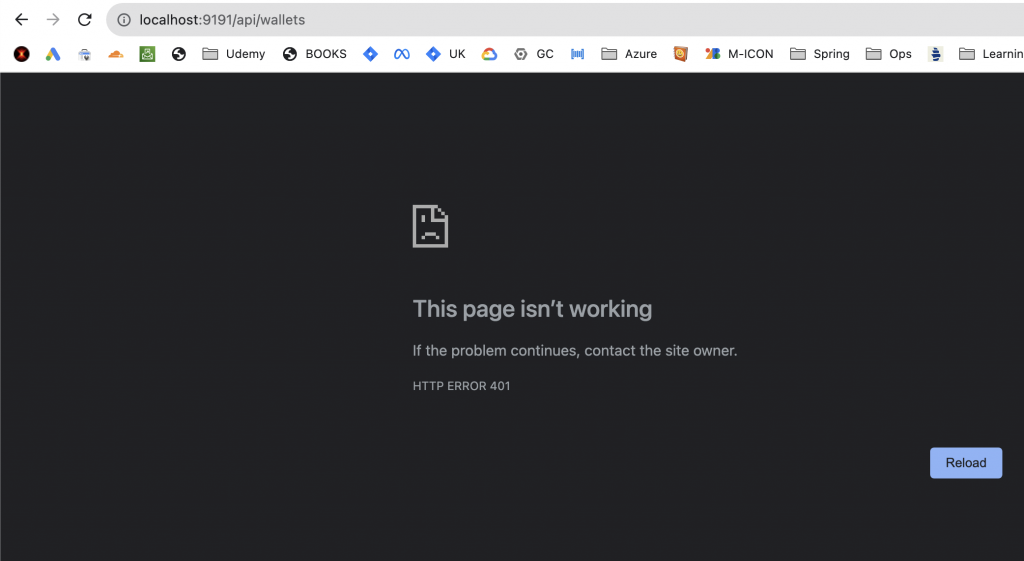
The backend behaves as we expected so far. Let’s try to grab a token from the frontend app and see if we can access the secured backend API with that token:
Obtain Auth0 token from the Angular app
Let’s open the Angular app and login. Make sure to open the dev tools. After logging in, you’ll see that there is a call to the token endpoint:
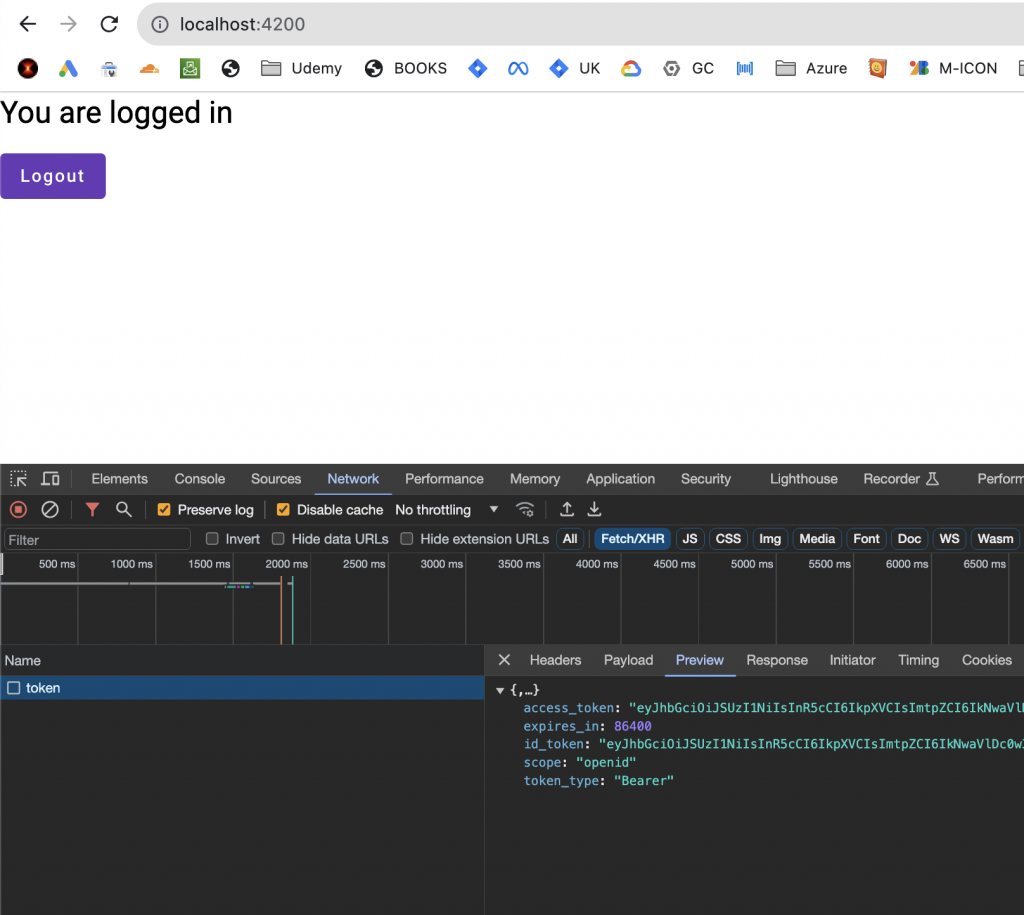
Let’s copy the access token and try to call the backend API using CURL:
curl -H "Authorization: Bearer eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6IkNwaVlDc0w3VHRvcGpzeW1PWHg5RyJ9.eyJpc3MiOiJodHRwczovL2Rldi12bXd3MzA1ZDdiMXVlNzU4LnVzLmF1dGgwLmNvbS8iLCJzdWIiOiJhdXRoMHw2NTJmZDkzNThiYzUzYmUyZDAxYmJhM2MiLCJhdWQiOlsiaHR0cDovL2xvY2FsaG9zdDo5MjkyIiwiaHR0cHM6Ly9kZXYtdm13dzMwNWQ3YjF1ZTc1OC51cy5hdXRoMC5jb20vdXNlcmluZm8iXSwiaWF0IjoxNjk3Njk2NDkxLCJleHAiOjE2OTc3ODI4OTEsImF6cCI6ImlTbFpoYjE0eUFVN2hRRUtnWmFZWERBMjJ2NFlDaE9yIiwic2NvcGUiOiJvcGVuaWQifQ.qyVBj7DVmqjkM1SKQAOINk4HZmoUbFV12C3GpQ7U_JSZkIIZT2NiKSdEwHyQPfuSkwIIYimoDFRx2-QUnL6HMX6MJ89PyX2kBKxmQ55aO2-YvZ7ugReyG01irAzWsqdxJgs5SVQCG7cV82h9xxQ71Gq1RUabfCVfIv8PzKK82pDOQe0qlTwGq1U92dkXoim3A7ZkNblXcRnNybLVjbAJpijZIK2YuHXhRvzWT9Hg_7gpfrH-DrckdkourIM_nqtNtb972tMs4QJHkAsjWxowXP5Gt2DDXBeg6nyED4hFWNXvhuyHwCGJFlwsMf1xnizh7ayvJM67g3a4tp2_XzMRfg" http://localhost:9191/api/wallets
Sure enough, the call is successful:
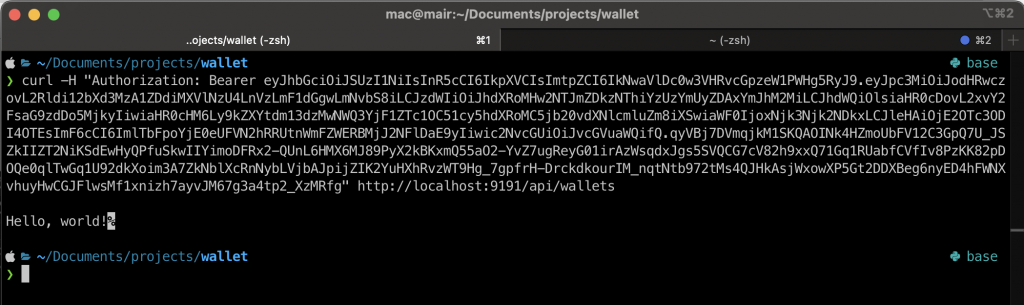
The integration with Auth0 is done. Now we can focus on our app.
Conclusion
In this post, we have tested our integration with Auth0 in both the backend and frontend applications. We was able to call the secured backend API using the bearer token obtained from the Angular app after logging in to Auth0. In the next post, we are going to setup some supporting services (MongoDB, HaProxy) before actually build the API.
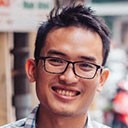
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.