Table of Contents
Introduction
In our previous post, we introduced the fundamentals of logging in Spring Boot, covering why logging is crucial and the basics of logging levels.
Now, we take a step further to explore how to customize your logging configuration. Spring Boot provides a flexible logging framework that can be easily adjusted to suit your needs, whether for development, testing, or production environments. In this post, we’ll cover how to configure logging levels and patterns for both console and file outputs in Spring Boot.
Configuring Logging Levels
Configuring logging levels in Spring Boot is straightforward, thanks to its use of application.properties
or application.yml
files. You can specify the logging level globally or for specific packages or classes, giving you fine-grained control over the amount of log output.
Global and Specific Logging Levels
To adjust the logging level globally or for a specific package/class, add the following lines to your application.properties
or application.yml
:
# Set global logging level logging.level.root=INFO # Set logging level for a specific package logging.level.org.springframework.web=DEBUG # Example for setting logging level for a specific class logging.level.com.datmt.logging=TRACE
logging: level: root: INFO org.springframework.web: DEBUG logging.level.com.datmt.logging: TRACE
Let’s add a service and create some logs using trace level.
package com.datmt.logging; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.stereotype.Component; import lombok.extern.slf4j.Slf4j; @SpringBootApplication @Slf4j public class LoggingTutorialApplication { public static void main(String[] args) { SpringApplication.run(LoggingTutorialApplication.class, args); } @Slf4j @Component public static class TestLogService { public TestLogService() { log.trace("Hello World"); } } }
When running the application, I can see the TRACE log from my package and DEBUG logs from Spring:
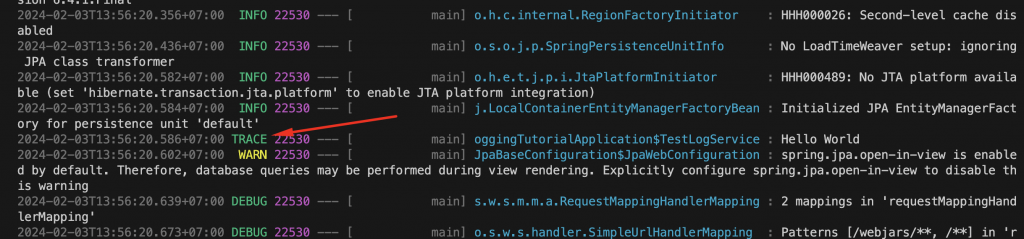
Console Logging Configuration
Console logging is often used in development environments for immediate feedback on the application’s behavior. Spring Boot allows customization of the console log format through patterns.
Customizing Console Log Pattern
You can customize the console log output by specifying a pattern in the logging configuration:
application.properties
logging.pattern.console=%d{yyyy-MM-dd HH:mm:ss} [%thread] %-5level %logger{36} - %msg%n
application.yaml
logging: pattern: console: '%d{yyyy-MM-dd HH:mm:ss} [%thread] %-5level %logger{36} - %msg%n'
This pattern includes the timestamp, thread name, log level, logger name, and the log message itself, providing a comprehensive view of the event.
File Logging Configuration
Logging to a file is essential for persisting log information for later analysis, especially in production environments. Spring Boot simplifies file logging configuration, including options for file rotation and maximum file size.
Basic File Logging
To log into a file, specify the file path and name in your configuration:
application.properties
logging.file.name=logs/application.log
application.yaml
logging: file: name: logs/application.log
Rolling Log Files
For applications with significant log output, you may want to use rolling log files to manage the size and number of log files:
Rolling Log Files
For applications with significant log output, you may want to use rolling log files to manage the size and number of log files:
application.properties
logging.logback.rollingpolicy.max-file-size=10MB logging.logback.rollingpolicy.max-history=10 logging.logback.rollingpolicy.total-size-cap=100MB
application.yaml
logging: logback: rollingpolicy: max-file-size: 10MB max-history: total-size-cap: 100MB
This configuration sets up a rolling file logger that creates a new log file when the current file reaches 10MB, keeps a maximum of 10 archived log files, and restricts the total size of log files to 100 MB.
10MB of log is a lot so I’ll set the file size to a lower value so you can see file logging with rolling in action. Let’s say the max file size is 1KB and the total size cap is 10KB.
logging.file.name=logs/datmt-logging.log logging.logback.rollingpolicy.max-file-size=1KB logging.logback.rollingpolicy.max-history=10 logging.logback.rollingpolicy.total-size-cap=10KB logging.logback.rollingpolicy.file-name-pattern=application.%d{yyyy-MM-dd}.%i.gz
I will also create a for loop to continuously print the log to the file.
@Slf4j @Component public static class TestLogService { public TestLogService() { while (true) { log.trace("Hello World"); } } }
As a result, when the application write logs:
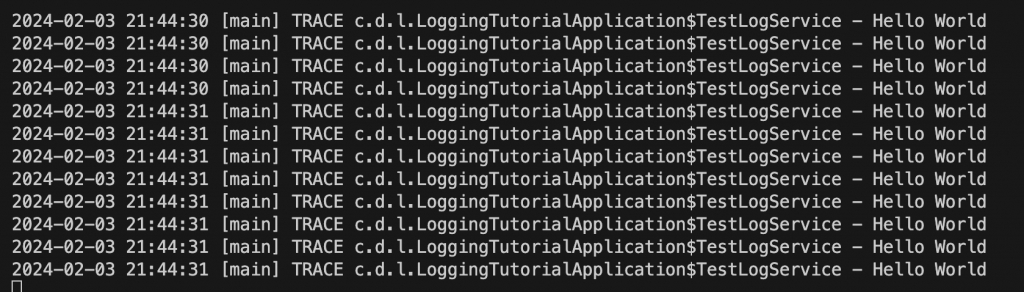
It also writes a log to a log file as I specified and compresses the log once the log file gets to a certain size (not necessarily the size I specified in the config).

Conclusion
Customizing logging in Spring Boot allows developers to tailor log output to their specific needs, whether to debug issues during development or monitor application behavior in production. By configuring logging levels and patterns for both console and file outputs, developers can ensure they have access to the right information at the right time. Stay tuned for our next post, where we will delve into advanced logging configurations, including log aggregation and monitoring in distributed systems.
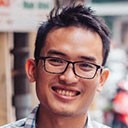
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.