Table of Contents
Introduction
Logging is an essential aspect of software development, providing insights into what’s happening inside an application running in development or production.
Effective logging practices help developers diagnose issues, understand application behavior, and improve performance.
Spring Boot simplifies the logging process, allowing developers to focus more on building features rather than configuring logging infrastructure.
This post introduces you to the fundamentals of logging in Spring Boot, highlighting its importance and how to leverage Spring Boot’s default logging capabilities.
Why Logging Matters
Logging serves multiple purposes in application development and maintenance:
- Debugging: Logs provide detailed insights into application flow and state, making it easier to identify and fix bugs.
- Monitoring: By analyzing logs, developers and operations teams can monitor application health, performance, and usage patterns.
- Audit and Compliance: Logs can be used to track user actions and system changes, aiding in security audits and compliance with regulatory requirements.
In simple terms, when things go wrong, the log is one of the most reliable (possibly the most reliable) source of information you have.
Spring Boot and Logging
Spring Boot uses Logback as its default logging framework, preconfigured for immediate use out of the box. Logback offers fast logging speeds, a simple configuration model, and powerful log management capabilities.
Spring Boot’s logging system is abstracted through the Spring Framework’s logging facade, which integrates with the Simple Logging Facade for Java (SLF4J) to provide a flexible logging API.
Understanding Logging Levels
Logging levels are used to categorize the importance of the log messages. Spring Boot supports the following levels (listed in order of increasing priority):
- TRACE: Provides the most detailed information. Suitable for diagnosing problems during development.
- DEBUG: Offers detailed debug information, less granular than TRACE.
- INFO: Provides informational messages that highlight the application’s progress.
- WARN: Indicates potentially harmful situations that are not necessarily errors.
- ERROR: Reports error events that might still allow the application to continue running.
- FATAL: Used to log very severe error events that will presumably lead the application to abort.
Developers can configure the logging level in Spring Boot applications to control the amount of logging information printed to the console or a file.
Configuring Logging Levels in Spring Boot
Spring Boot allows developers to configure logging levels in the application.properties
or application.yml
files, enabling easy adjustments without changing the code. Here’s how you can set the logging level for your entire application or specific packages:
application.properties
# Set global logging level logging.level.root=INFO # Set logging level for a specific package logging.level.org.springframework.web=DEBUG
application.yaml
logging: level: root: INFO org.springframework.web: DEBUG
Conclusion
Understanding the basics of logging in Spring Boot is crucial for effective application development and maintenance. By leveraging Spring Boot’s default logging framework and configuring logging levels appropriately, developers can gain valuable insights into their applications’ behavior and health. Stay tuned for our next post, where we’ll dive deeper into configuring logging outputs in Spring Boot, including console and file logging.
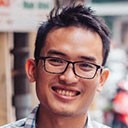
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.