Table of Contents
Introduction
What is a database connection pool
A database connection pool is a cache of open database connections that can be used by any thread that needs to access a database. Instead of creating a new connection each time a thread needs to access the database, the thread can borrow a connection from the pool and use it to execute the necessary queries. When it is finished, it can return the connection to the pool so that it can be used by another thread.
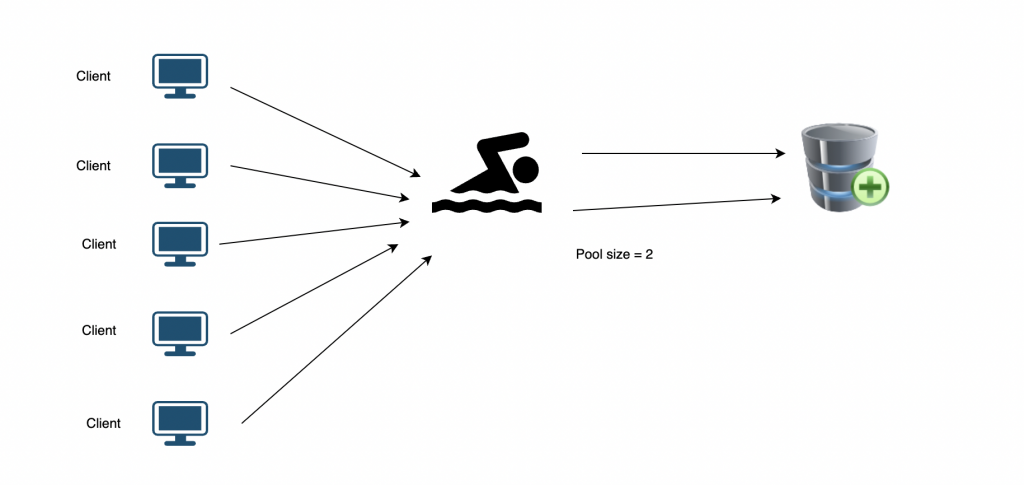
Why connection pools are useful for Java applications
Connection pools are useful for Java applications because they can help improve the performance of the application by reducing the overhead of creating and destroying connections each time they are needed. Creating a new connection to a database can be a time-consuming process, especially if the database server is located on a remote network. By using a connection pool, multiple threads can share a smaller number of connections, reducing the need to create new connections and improving the overall performance of the application.
When a thread needs a connection but none is available, what happens?
When a thread requests a connection from a connection pool, but there are no connections available in the pool, the behavior will depend on the configuration of the connection pool manager. In some cases, the thread may be blocked until a connection becomes available. In other cases, the connection pool manager may throw an exception to indicate that no connections are available.
For example, if the connection pool manager is configured with a maximum wait time, the thread will be blocked until a connection becomes available or the maximum wait time is reached. If the maximum wait time is reached, the connection pool manager will throw a TimeoutException
to indicate that no connections are available.
Alternatively, if the connection pool manager is configured to not block when no connections are available, it will immediately throw a SQLException
to indicate that no connections are available.
Overall, the specific behavior when a thread requests a connection but none is available will depend on the configuration of the connection pool manager. It is important to understand the configuration options and choose the appropriate settings for your application.
How connection pools work
Let’s dive into the mechanism of connection pool management.
How connections are created and managed
Connections in a connection pool are typically created when the connection pool manager is initialized. The connection pool manager will create a number of connections and store them in the pool, ready to be used by any thread that needs to access the database.
When a thread requests a connection from the pool, the connection pool manager will check the pool for an available connection. If a connection is available, it will be borrowed by the thread and used to execute the necessary queries. When the thread is finished, it will return the connection to the pool.
The connection pool manager is responsible for managing the connections in the pool, including ensuring that there are always enough connections available to meet the needs of the application, closing idle connections to prevent them from timing out, and creating new connections if necessary to maintain the desired pool size.
How to determine the optimal pool size?
Determining the optimal pool size for a connection pool is not a simple task, and there is no one-size-fits-all solution. The optimal pool size will depend on a number of factors, including the number of concurrent threads that need to access the database, the maximum number of connections allowed by the database server, the performance and latency of the database, and the workload of the application.
To determine the optimal pool size, it is often necessary to experiment with different pool sizes and monitor the performance of the application. By measuring the response time and throughput of the application with different pool sizes, you can determine the pool size that provides the best balance between performance and resource utilization.
In general, a larger pool size will provide better performance by reducing the overhead of creating and destroying connections, but it will also consume more resources and may not be necessary if the workload of the application does not require it. On the other hand, a smaller pool size will consume fewer resources, but it may not be able to handle the workload of the application if the number of concurrent threads is high.
Overall, determining the optimal pool size for a connection pool is a balancing act that requires careful consideration of the specific needs and workload of the application.
Types of connection pool managers for Java
Here are ones of the most common connection pool managers in Java
- Apache DBCP: Apache DBCP is a widely used open-source connection pool manager that is known for its simplicity and reliability. It provides basic features for creating and managing connections in the pool, such as configurable pool sizing, connection validation, and connection leak detection.
- C3P0: C3P0 is another widely used open-source connection pool manager that offers more advanced features, such as support for data sources and JNDI. It also provides support for connection testing, statement caching, and transaction management.
- HikariCP: HikariCP is a relatively new but quickly growing connection pool manager that is known for its high performance and low memory footprint. It provides a number of advanced features, such as support for the Java 8 CompletionStage API, and the ability to fine-tune the performance of the connection pool through a number of configurable settings.
Overall, each of these connection pool managers has its own strengths and weaknesses, and the right choice will depend on the specific needs of the application.
Monitoring and managing a connection pool
When monitoring a connection pool, there are several metrics that are important to consider, including:
- Active connections: The number of connections that are currently in use by threads. A high number of active connections may indicate that the pool is unable to keep up with the workload of the application, or that the pool size is too small.
- Idle connections: The number of connections that are currently not in use by threads. A high number of idle connections may indicate that the pool size is too large, and that the application is not using all of the connections in the pool.
- Connection wait time: The amount of time that threads have to wait for a connection to become available in the pool. A high connection wait time may indicate that the pool is unable to provide connections quickly enough to meet the needs of the application.
- Connection timeout: The amount of time that a connection is allowed to remain idle in the pool before it is closed by the connection pool manager. A high connection timeout may indicate that the pool is not being used efficiently, and that connections are being closed prematurely.
Overall, these are some of the key metrics to consider when monitoring a connection pool. By monitoring these metrics, you can gain insight into the performance of the pool, and identify potential issues that may need to be addressed.
Conclusion
The post provides you a basic introduction of the concept of connection pools (in Java). In the future post, I’m going to give you some hands-on experience working in connection pools in Java applications (mostly Spring Boot).
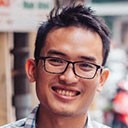
I build softwares that solve problems. I also love writing/documenting things I learn/want to learn.